Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial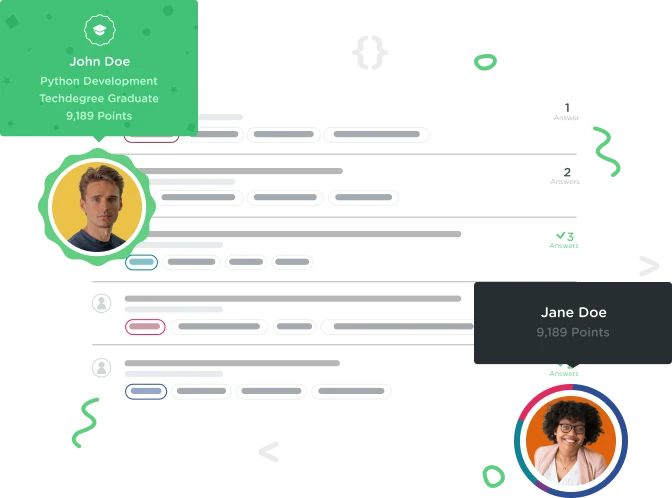
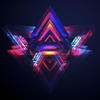
Krunal Patel
1,770 PointsI am getting undefined when showing the questions.
unction print(message) {
document.write(message);
}
var quiz = [
[' What color is the circle on the Japanese national flag?','red'],
[' What is the chemical symbol for Hydrogen?','h'],
[' How many sides does an octagon have?','8'],
[' Great Whites and Hammerheads are what type of animals?','shark']
]
function test(choice){
var count = 0;
for (var i = 0; i < choice.length; i++){
var question = prompt(choice[i][0]);
question = question.toLowerCase();
var right;
var wrong;
var answer = question;
if ( question === choice[i][1] || question === 'eight'){
right+= `<ul><li>${choice[i][0]}</li></ul> \n ${answer}`;
count++;
} else {
wrong+= `<ul><li>${choice[i][0]}</li></ul> \n ${answer}`;
}
}
print(`You got ${count} question(s) right<br>`);
print(`<h1>The correct questions you got are</h1> ${right}`);
print(`<h1>The wrong questions you got are</h1> ${wrong}`);
}
test(quiz);
3 Answers
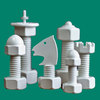
Steven Parker
231,269 PointsThe first time you use the concatenating assignment operator ("+=") on your "right" and "wrong" variables, their initial state is "undefined". To fix that, just initialize them to the empty string:
var right = "";

kevin hudson
Courses Plus Student 11,987 PointsThe variable right should be initialized to zero and I think it's best outside of the loop to put all your global scope variables up top for easier reading and understanding.
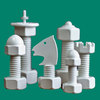
Steven Parker
231,269 PointsI agree that it's "best practice" to group globals together at the beginning, but you would not want to initialize the "right" and "wrong" variables with 0, because that would cause a "0" to appear on the page in front of the lists that will be concatenated onto them later. It's best to start them as empty strings.

kevin hudson
Courses Plus Student 11,987 PointsYes, in this case, it would be since he is adding a string to right and wrong variable. My explanation was to utilize them as counters and I overlooked the code so yes you are right. I noticed other mistakes along the way where this wouldn't work. the variable question was being changed along the way and I made some changes so you can see where mistakes were made. Mistakes are good because you can learn from correcting them. Take time to digest the code below. This works but there are better ways to keep it DRY (don't repeat yourself) and more readable for others.
function print(message) {
document.write(message);
}
var quiz = [
[' What color is the circle on the Japanese national flag?','red'],
[' What is the chemical symbol for Hydrogen?','h'],
[' How many sides does an octagon have?','8'],
[' Great Whites and Hammerheads are what type of animals?','shark']
]
function test(choice){
var count = 0;
var right ='';//initialize here
var wrong = '';
for (var i = 0; i < choice.length; i++){
var question = prompt(choice[i][0]);
var answer = choice[i][1];// moved answer to value of array
if ( question === answer){// makes more sense
// you can assign the question part [i][0] to a variable instead of call out the array elements below
right+= `<ul><li>${choice[i][0]}</li></ul> \n ${answer}`; // now answer works
count++;
} else {
wrong+= `<ul><li>${choice[i][0]}</li></ul> \n ${answer}`;
}
}
print(`You got ${count} question(s) right<br>`);
print(`<h1>The correct questions you got are</h1> ${right}`);
print(`<h1>The wrong questions you got are</h1> ${wrong}`);
}
test(quiz);