Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial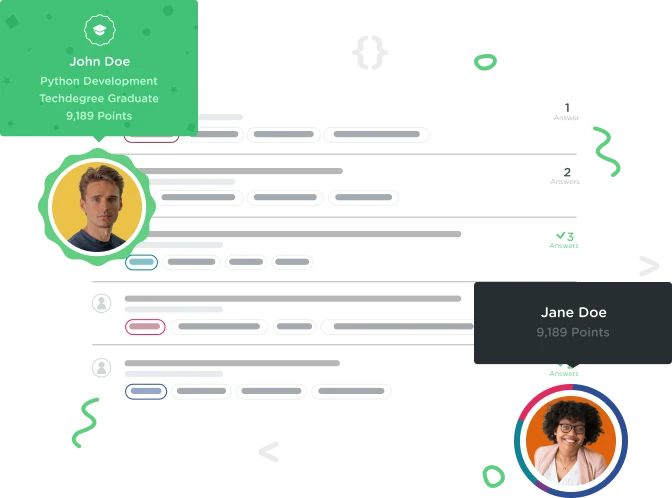

Kaleb Ross
4,868 PointsI am having a hard time understanding what to put down in do while. Any suggestions?
Not sure how I get it to do what I want to do. I have re wrote it several times.
var secret = prompt("What is the secret password?");
do {
console.log(
}
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
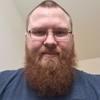
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHave a look at this:
do {
// do something here
} while (condition);
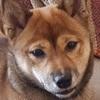
Katie Wood
19,141 PointsHi there,
You generally have the right idea - the main thing to remember is that, in a do-while, there is no loop code after the while() condition - all of the code goes between "do" and "while". Henrik's answer shows the basic syntax really well. It looks like you want it to keep asking for the password until the user enters "sesame" - if you're stuck, here's an example of how you would write your current code into a valid do-while loop:
var secret = '';
do {
var secret = prompt("What is the secret password?");
} while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
We just initialize the variable at the top - we don't need to prompt yet, because the loop will do that for us. When it gets through the loop, it will check the 'while' condition - if the user did not enter 'sesame', it will loop back through and prompt again.
Does that make sense? Happy coding!
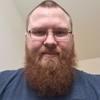
Henrik Christensen
Python Web Development Techdegree Student 38,322 Pointsvar secret = ''; // here you create the variable "secret"
do {
// by saying "var secret" here you create a NEW variable named secret
// this is a totally different variable than the variable at the top
var secret = prompt("What is the secret password?");
} while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
// -----
var secret;
do {
secret = prompt("What is the secret password? ");
} while (secret !== "sesame");
docoment.write("You know the secret password. Welcome.");

Kaleb Ross
4,868 PointsThank you both...I am going to have to review the videos again. I am having problems still even with both of your help. Thank you
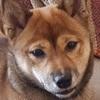
Katie Wood
19,141 PointsI'll break it down a little more here, just in case it helps. I'm going to write a do-while loop that will ask the user if they want to keep looping. If they say "no", it stops, but otherwise it will keep asking. I'll space things out so it will hopefully be easy to see what code goes where.
var input = ''; //this is where we'll store the user's input - no value for now
do {
input = prompt("Do you want to keep looping?");
} while (input !== "no")
A do-while loop can basically be read as "do {this code} while (this condition is accurate)". So, this one is 'Do a user prompt asking if the user wants to keep looping while their input is not "no"'. In a do-while loop, the code inside will always be executed once - it won't check the while condition until after it goes through once. Then, it will check after each loop.
In the above example, it will prompt the user once and save the value in the input variable. Then, it will compare the variable to "no" - if they are not equal, it will prompt again and update the value. Then, it will compare again, and so on.
Does that make sense? I hope reviewing the videos helped - let me know if there's anything else I can do.