Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial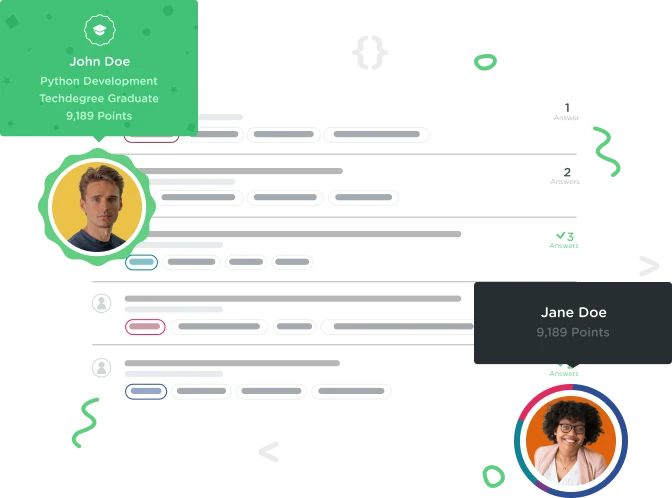
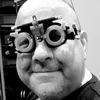
Trevor Codner
1,700 PointsI am having an issue with an If / elif block, can anyone advise me where i might be going wrong?
I have made a body weight calculator just for practice, it seems to work fine except in the if elif block near the end it always prints out the man or male reply, never the woman / femail string. You will note the two strings that don't seem to work as I have added male and femail to the output to make it clear which one is printing, seems always to be male.
I have run it interactively and have checked that the gender variable get populated ok. I am now officially scratching my head............
HELP
# Get Age & store in a variable
age = int(input("What is your age in years ? "))
# Get persons Gender
gender = str(input("Are you a man or a woman ? "))
# get weight kgs and store
weight = float(input("What is your weight in Kilo's ? "))
# Get height bmi_target = int(input("what is your target BMI"))
height = float(input("What is your height in metres? (Example 1.75 ) "))
# calculate BMR here and store in a variable
bmr_man = (10 * weight) + 6.25 * height - (5 * age) + 5
bmr_woman = (10 * weight) + (6.25 * height) - (5 * age) + 161
# calculate BMI here and store in a variable
bmi = (weight / height) / height
# Round the BMI result down to 1 decimal place
bmi = str(round(bmi)) # This was the original ** bmi = str(round(bmi), 1) the output exaple was 29.9 and the .9 was of no use
# Round the BMI result down to 1 decimal place
bmr_man = str(round(bmr_man))
bmr_woman = str(round(bmr_woman))
print() # adds a blank line to aid formating
if gender.lower == "man" or "m" or "male":
print("MALE You require " + bmr_man + " Calories just to maintain your current body weight ")
elif gender.lower == "woman" or "w" or "f":
print("FEMALE You require " + bmr_woman + " Calories just to maintain your current body weight ")
else: print("Your BMR is: Unknown")
print("BMR is a measurement of the calories you require each day, based on staying in bed or doing nothing")
print() # adds a blank line to aid formating
if float(bmi) <= 18:
print("Your Body Mass Index of " + bmi + " Suggests you are Underweight ")
elif float(bmi) >= 18.5 and float(bmi) <= 24.9:
print("Your Body Mass Index of " + bmi + " Suggests you are Healthy ")
elif float(bmi) >= 25 and float(bmi) <= 29.9:
print("Your Body Mass Index of " + bmi + " Suggests you are Overweight ")
elif float(bmi) >= 30 and float(bmi) <=39.9:
print("Your Body Mass Index of " + bmi + " Suggests you are Obese ")
else:
print("Your Body Mass Index of " + bmi + " Suggests you are Morbidly Obese ")
2 Answers
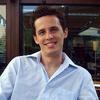
Pedro Cabral
33,586 Pointsif gender.lower == "man" or "m" or "male":
This doesn't work as you think. In fact, it evaluates always to true because of the or "m" since "m" is not an empty string it returns true right there. To fix it, you should write if foo == a or foo == b or foo == c meaning, that you have to evaluate a "condition" in all your ors/ands.
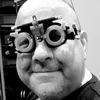
Trevor Codner
1,700 PointsThank you Pedro, for pointing that out, I just could not see it. Will try it when I get home.
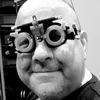
Trevor Codner
1,700 Pointsstill having an issue with this code, spent three hours last night and a couple of hours this morning trying to figure out why the if block is running through to: "your BMR is unknown".
I have but a few lines of test code in to show where calculation are being done and variables stored. I even ran the code through python visualiser and there are no errors, it just appear that gender does not match anything in the if statement.
Here is the code: PS: I must be missing something really obvious...... This is I think the offending code:
if gender.lower == "m" or gender.lower == "male":
print("M You require " + bmr_man + " Calories just to maintain your current body weight ")
elif gender.lower == "f": # or gender.lower == "female":
print("F You require " + bmr_woman + " Calories just to maintain your current body weight ")
else: print("Your BMR is: Unknown")
& this is the full code (sorry to be a pain)
# Purpose - Basal Metabolic Rate -
# Get Age & store in a variable
age = int(input("What is your age in years ? "))
# Get persons Gender
gender = input("Are you m or f ? ") # inputs are already a string so no need to coerce.
# get weight kgs and store
weight = float(input("What is your weight in Kilo's ? "))
# Get height bmi_target = int(input("what is your target BMI"))
height = float(input("What is your height in metres? (Example 1.75 ) "))
# calculate BMR here and store in a variable
bmr_man = (10 * weight) + 6.25 * height - (5 * age) + 5
bmr_woman = (10 * weight) + (6.25 * height) - (5 * age) + 161
# Round the BMI result down to 0 decimal place
bmr_man = str(round(bmr_man))
bmr_woman = str(round(bmr_woman))
# calculate BMI here and store in a variable
bmi = (weight / height) / height
# Round the BMI result down to 1 decimal place
bmi = str(round(bmi)) # This was the original ** bmi = str(round(bmi), 1) the output exaple was 29.9 and the .9 was of no use
print() # adds a blank line to aid formating
print("This is just test code to prove the variables are being populated") #
print("-----------------------------------------------------------------")
print("Test Gender entered was: " + gender) # test line to prove gender is stored ok
print("Test bmr for a man is: " + bmr_man) # test line to prove bmr_man is being calculated & stored
print("Test bmr for a woman is: " + bmr_woman) # test line to prove bmr_woman is being calculated & stored
print() # adds a blank line to aid formating
if gender.lower == "m" or gender.lower == "male":
print("M You require " + bmr_man + " Calories just to maintain your current body weight ")
elif gender.lower == "f": # or gender.lower == "female":
print("F You require " + bmr_woman + " Calories just to maintain your current body weight ")
else: print("Your BMR is: Unknown")
print("BMR is a measurement of the calories you require each day, based on staying in bed or doing nothing")
print() # adds a blank line to aid formating
if float(bmi) <= 18:
print("Your Body Mass Index of " + bmi + " Suggests you are Underweight ")
elif float(bmi) >= 18.5 and float(bmi) <= 24.9:
print("Your Body Mass Index of " + bmi + " Suggests you are Healthy ")
elif float(bmi) >= 25 and float(bmi) <= 29.9:
print("Your Body Mass Index of " + bmi + " Suggests you are Overweight ")
elif float(bmi) >= 30 and float(bmi) <=39.9:
print("Your Body Mass Index of " + bmi + " Suggests you are Obese ")
else:
print("Your Body Mass Index of " + bmi + " Suggests you are Morbidly Obese ")
Trevor Codner
1,700 PointsTrevor Codner
1,700 Pointshmmmm how do i post code? Sorted