Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial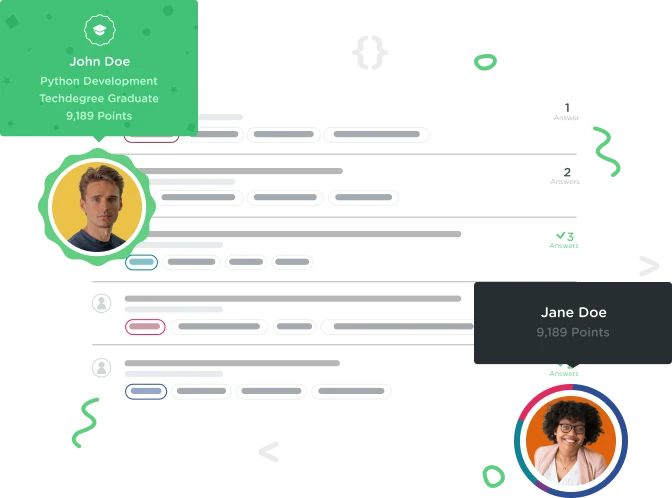

Trace Harris
Python Web Development Techdegree Graduate 22,065 PointsI am having some issues with Unit testing:
#undertest
def userchoice1():
"""
grabs and validates user choice
Initialize menu object to display menu items variable menu1 prompts for
and stores user input while loop runs until user provides a valid input
based upon input the method calls: either self.user_entry_data method
or will call an instance of menu.submenu() which is a method of
Menu class
"""
# grab and store user input
menu = Menu()
menu.main()
while True:
menu1 = input(
'please choose '
'option a), or '
'option b) '
)
# tests for a valid resonse
# if user provides valid response loop will break
if menu1.lower() not in ('a', 'b'):
print("Not an appropriate choice.")
else:
break
# based upon user input call method
if menu1 == 'a':
clear()
main = Main()
main.user_entry_data(*data_collection())
elif menu1 == 'b':
menu.submenu()
sub = Main()
sub.user_search()
#___________________________________________________
#testsuite
def test_user_choice(self, Mockinput):
#so I creating a sideeffect to see if 'a'
Mockinput.side_effect =['a']
expected_output = main.userchoice1()
#var that will be passed to return value
returnvalue = ('ted',
utilities.datetime(1999, 12, 23, 0, 0),
'frank',
utilities.timedelta(seconds=7380),
'somenotes'
)
#trying an objectpatch to mock function with my return values
with patch.object(main, 'main.data_collection', return_value=returnvalue, side_effect=None) as mock:
expected_output = main.userchoice1()
mock.assert_called_once_with(returnvalue)
Trace Harris
Python Web Development Techdegree Graduate 22,065 PointsTrace Harris
Python Web Development Techdegree Graduate 22,065 PointsSo I am trying to come up with a Testing strategy for this function, currently I am patching builtins.Input using the
unittest.mock' library. here are some behaviors of the function takes a input if
input= 'a' So Ideally I would want ifa: main.main.user_entry_data assert called once
elseMenu.submenu
called once