Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial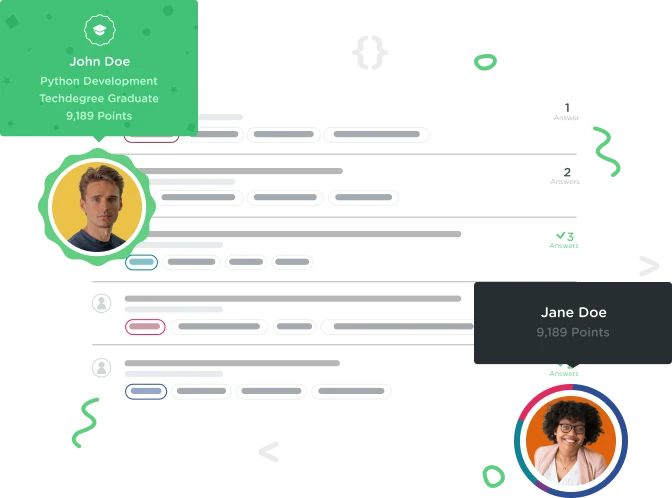

Chiroi Niehus
6,729 PointsI Am Having Trouble on Code Challenge: Chance Scoring Task 1 of 2
I am stuck on this code challenge. This whole Object-Oriented course has been very confusing in general, but this whole Yatzy section in specific is hard to understand. On this challenge, I tried to return the sum of the argument 'hand', but it gives me the error:
Bummer: TypeError: score_chance() takes 1 positional argument but 2 were given
I am not sure why it is giving me this, since I clearly only gave the function one argument. Please help!
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(hand):
return sum(hand)
2 Answers

Brett Miller
3,663 PointsHello Chiroi,
This can be a confusing error to get. The short answer is that you need to pass self in as the first parameter in the score_chance method. For a little more detail:
# Create a new instance
my_scoresheet = YatzyScoresheet()
# You would call your method like this on your instance
my_scoresheet.score_chance(hand)
# Behind the scenes though python calls this
YatzyScoresheet.score_chance(my_scoresheet, hand)
So your error "TypeError: score_chance() takes 1 positional argument but 2 were given" is telling you that the method you created in your example only takes 1 argument (hand) but is being passed 2 arguments (instance, hand) when called behind the scenes in the challenge. Here is a stackoverflow post explaining it: TypeError: method() takes 1 positional argument but 2 were given
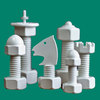
Steven Parker
230,274 PointsAs Brett pointed out, you defined the method with one argument but it needs two.
Remember that all instance methods take "self" as the first argument. Look at the "score_one_pair" method for an example.