Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial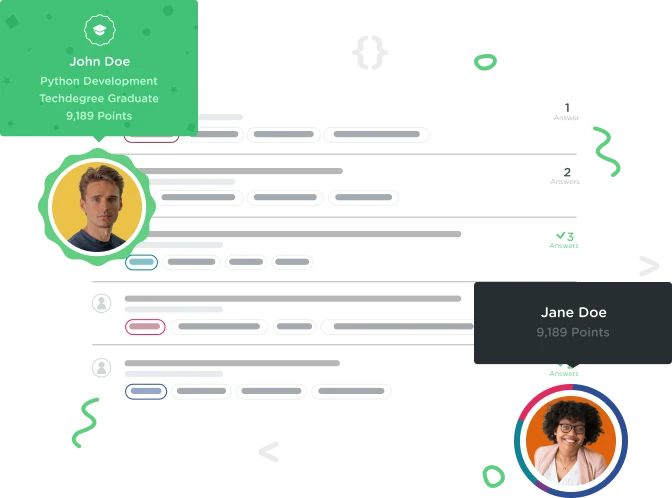

John Fouts
12,234 PointsI am having trouble understanding what I need to do in order to pass the 2nd part of this challenge. Please help.
I have been trying to pass the second portion of this challenge for some time. I have tried many different versions of methods from Stack Overflow and various sources on the Internet. Unfortunately, I am unable to pass this challenge. Can you please help?
// Initializing Using Statements
using System;
// Establish Calculator Type
public class Calculator
{
public double Result{get;set;}
public Calculator(double Result)
{
Result = 0.0;
}
private double m_Total;
public double Add(double value)
{
m_Total += value;
return m_Total;
}
// Initialization Class To Establish Value of Calculator
// public void Initialization()
// {
// Calculator calculator = new Calculator(1.1);
// }
//
// public Calculator(double val)
// {
// var newCalc = 1.1;
//Calculator(newCalc);
//return keyword must not be followed by any expression when method returns void
//Compilation failed: 1 error(s), 0 warnings
// }
// public double Result{get;set;}
// public void Add(double val)
// {
// throw new NotImplementedException();
// }
//Here, you want them to be "implemented".
//For the purposes of testing, you want the functions
//implemented but empty. Don't throw the exceptions,
//that's essentially the same as not having the function at all.
// Bummer! Assert.Equal() Failure, Expected: 1.1 (rounded from 1.1), Actual: 0 (rounded from 0)
// Initialize Calculator
// Square function
//public double Square(double num)
//{
//return num*num;
//}
// Add two doubles and returns the sum
//public double Add(double num1, double num2 )
//{
//return num1 + num2;
//}
// Multiply two integers and retuns the result
//public double Multiply(double num1, double num2 )
//{
//return num1 * num2;
//}
// Subtracts small number from big number
//public double Subtract(double num1, double num2 )
//{
// if ( num1 > num2 )
// {
// return num1 - num2;
// }
// return num2 - num1;
//}
//public double Result(double num1)
//{
//}
// CalculatorTests.cs(19,16): error CS1502: The best overloaded method match for `Xunit.Assert.Equal(double, double, System.Collections.Generic.IEqualityComparer)' has some invalid arguments
///usr/local/lib/ruby/gems/2.3.0/gems/challenge_proctor-0.0.0/lib/challenge_proctor/runners/includes/mono/xunit.assert.dll (Location of the symbol related to previous error)
//CalculatorTests.cs(19,39): error CS1503: Argument `#2' cannot convert `method group' expression to type `double'
//Compilation failed: 10 error(s), 0 warnings
//
}
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
}
3 Answers
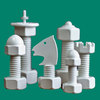
Steven Parker
230,688 PointsYou might be confusing yourself.
Not that resources like "Stack Overflow" aren't valuable (they are!), but they could over-complicate the generally simple solutions needed for the course challenges. But issues I noticed with your code:
- you gave your constructor parameter the same name as your class property
- your constructor takes a parameter but does not use it (it should probably assign it to the property)
- your Add routine uses a private field instead of the public property
- the private field isn't needed, based on the functionality implied by the tests

John Fouts
12,234 PointsSteven - I appreciate your support more than you know! - as you have helped me out tremendously on some other challenges. On this one, however I had already arrived at a solution before reading the guidance. You did definitely provide some solid pointers here too. Why I didn't select yours as the best answer originally is because you said that resources like Stack Overflow aren't valuable. Thank you for your guidance and for the excellent tips.
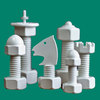
Steven Parker
230,688 PointsSorry if I confused things with the double negative. I said "Not that resources like "Stack Overflow" aren't valuable"... which means that they are valuable. In fact, I refer to "Stack Overflow" quite frequenty myself — just not for Treehouse course challenges.

John Fouts
12,234 Pointspublic Calculator(double val) { Result = val; }
public double Result {get; set; }
public double Add (double val) { return Result += val; }
public double Subtract(double val) { return Result += -val; }
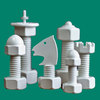
Steven Parker
230,688 PointsLeaving code solutions (known as "spoilers") might not be the best way to help other students learn. That's why I generally take extra time to analyze the issues in a question and provide suggestions and hints.
Give a man a fish and you feed him for a day; teach a man to fish and you feed him for a lifetime.
― Maimonides

John Fouts
12,234 PointsSteven - the double negative did not confuse me. I had my five year old tearing at my arm when I was trying to complete this. I could almost swear the wording was different previously, but I have been really tired so I am sure it was just me. I must have read it as Note... is all I can think. At any rate, thank you for clearing that up. With that being said, I wish that I could change the best answer to your answer. Thank you again for all of your support regarding the ongoing challenge/solution Treehouse course quizzes. It means a lot to me as a student going through the courses on Treehouse that people like you are available as resources to help students along the way. I have experience coding in several languages (Fortran, Pascal, VB, QBasic, VBA...), but I am very rusty having not coded for a while (although I did take a course a couple of years ago where I started learning HTML/CSS/Java Script and a small part of jQuery - it was super exciting! I have enjoyed getting back into coding and learning c#/.NET! Throwing MVC into the mix has been very challenging for me to learn, but I keep plugging away, and as a student I want to tell you thanks again for being there as a guide and as a mentor to help those of us that get a little puzzled at times.
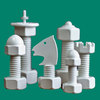
Steven Parker
230,688 PointsIt looks like you were successful in resetting your best answer choice (it should always be possible to do that) .. or perhaps some mod passed by and helped out.
John Fouts
12,234 PointsJohn Fouts
12,234 PointsI figured it out before I saw this actually, and yes I was confusing myself. After trying so many different things and having them not work I was making this way more difficult than it needed to be. The Unit Testing course (in my opinion) probably because I am still new to the c# language, could have used a few more code challenges to help me out.
I ended up changing the Calculator.cs file to:
public class Calculator {
}
I went ahead and listed the Subtract method here in case others are having difficulty with this question too. I can see how confused I really was by reviewing the code I submitted earlier. Thanks for sending the information you sent - it is appreciated. Treehouse really is a good resource for learning how to code. I wish I had more time to absorb the information and content in each section. With experience more of the knowledge and content explained in the videos will become more solidified in my mind. Thanks again for your help.