Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial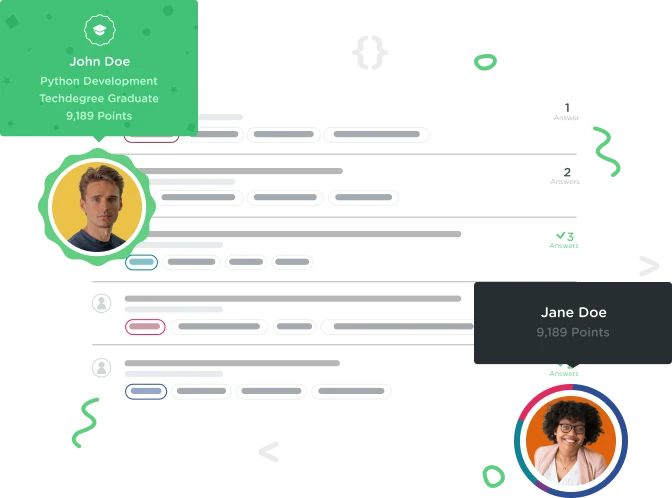

Alex Nelsen
Courses Plus Student 2,977 PointsI am having trouble with class and super class in their challenge.
I believe I have the majority of this correct but I am not sure. Please have a look.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName )
func someDoctor() ->String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(lastName: "Smith")
1 Answer

Michael Reining
10,101 PointsHi Alex,
The answer is actually a lot simpler than the code that you provided.
All you have to do in the new Doctor class is to override the getFullName method. You do not need to create a custom initializer since it will just inherit the initializer from the super class.
As always, if you try to create the code in the Playground in Xcode you will get more helpful error messages and see what is happening as you change the code. Really an excellent way to learn and makes code challenges much easier to solve.
Here is the code
// Enter your code below
class Doctor: Person { // Create new class
override func getFullName() -> String { // Override method
return "Dr. \(lastName)" // Update return string
}
}
let someDoctor = Doctor(firstName: "Mike", lastName: "Reining")
someDoctor.getFullName() // for testing in playground you can see that it works
I hope that helps,
Mike
PS: If you found the above helpful, be sure to check out my app which breaks code challenges down and really explains things so you can learn faster.
Code! Learn how to program with Swift
https://itunes.apple.com/app/code!-learn-how-to-program/id1032546737?mt=8
PPS: I could not have developed the above app without going through the awesome resources on Team Treehouse. Stick with it. It is so worth it!