Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial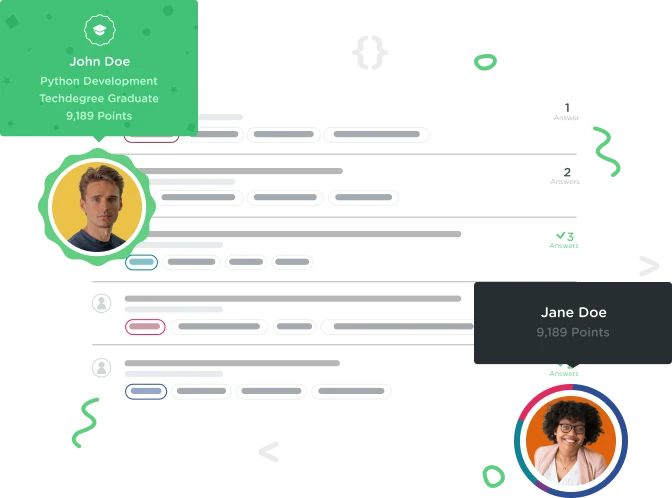

Benjamin Cohen
Full Stack JavaScript Techdegree Student 6,045 PointsI am having trouble with the shuffle the deck challenge, I've been working on it for quite some time.
function createDeck() {
deck = []
var suites = ['♠︎','♣︎','♥︎','♦︎'];
var ranks = ['Ace','King','Queen','Jack','10','9','8','7','6','5','4', '3','2'];
// add your code below here:
for (var i = 0; i < suites.length; i += 1){
for (var j = 0; j < ranks.length; j += 1){
var card = [ranks[j],suites[i]];
deck += card;
/*console.log(card); The output of this line is what I want my array to look like
*/
}
} return shuffle(deck);
console.log(shuffle(deck));
console.log(deck);
}
createDeck();
1 Answer

Tabatha Trahan
21,422 PointsOne way to solve this is to use the push method to add the result of your inner and outer loop to the deck array:
for(var i = 0; i < suites.length; i++){
for(var j = 0; j < ranks.length; j++){
deck.push([ranks[j], suites[i]]);
}
}
So within the inner loop, you are taking the rank and suite and putting them within an array, and then adding them to the main deck array. Then you can create a new deck that takes the results of the function you just created and print them out with another for loop:
var myDeck = createDeck();
for(i = 0; i < myDeck.length; i++){
console.log(myDeck[i].join(' of '));
There may be a better way to do this, but this is what I did to complete the challenge.
Benjamin Cohen
Full Stack JavaScript Techdegree Student 6,045 PointsBenjamin Cohen
Full Stack JavaScript Techdegree Student 6,045 PointsThank You Tabatha! Thanks for the answer and for the explanation as well.