Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial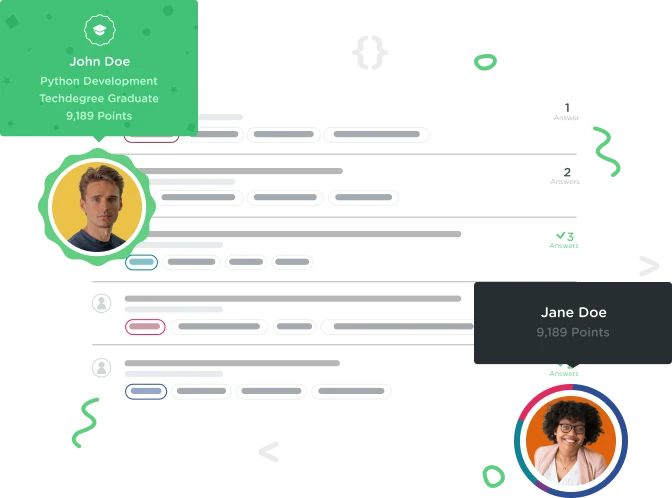

Shannon Ferguson
1,221 PointsI am hung up on this question.
Here is a multi-dimensional list of musical groups. The first dimension is group, the second is group members.
Can you
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"]
]
for item in musical_groups:
print(", ".join(musical_groups[item]))
3 Answers
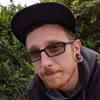
Travis Alstrand
Treehouse Project ReviewerHey there Shannon Ferguson !
Since we are already looping through each 'item' inside of musical_groups
, we don't need to specify it's placement within that list.
Just telling it to print the current 'item' it's looping across should do the trick
for item in musical_groups:
print(", ".join(item))
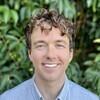
Asher Orr
Python Development Techdegree Graduate 9,409 PointsHi Shannon! This is the syntax for the .join method:
string.join(iterable)
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"],
]
for item in musical_groups:
print(", ".join(musical_groups[item]))
In your current code, you're iterating through each item. That's your iterable- if you replace what's in the parentheses after join with item (your iterable), it should pass the challenge.
I hope this helps. Let me know if you have any questions!
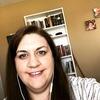
Nicole Groenewald
1,031 PointsWhen I do this code I get "Traceback (most recent call last): File"/home/treehouse/workspace/music.py", line 12 in <module> print(", ".joinmusical_groups[groups])) TypeError: list indices must be integers or slices, not list
Here's all my code, just in case
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"],
]
# Your code here
for groups in musical_groups:
print(", ".join(musical_groups[groups]))
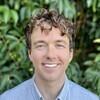
Asher Orr
Python Development Techdegree Graduate 9,409 PointsWhen you enter this code:
for groups in musical_groups:
You are looping through each list within musical_groups. "groups" essentially means "each list within musical_groups."
Each list within musical_groups has several strings (the members of the band/group.) To pass the challenge, you need to join the strings together.
Remember, this is the syntax for the .join() method:
string.join(iterable)
for groups in musical_groups:
string.join(iterable)
"groups" is your iterable.
print(", ".join(musical_groups[groups]))
A list index can't be another list- that type of syntax doesn't work in Python. That's why you're getting the TypeError.
Does this answer your question?
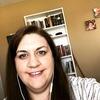
Nicole Groenewald
1,031 Pointsyes, thanks!