Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial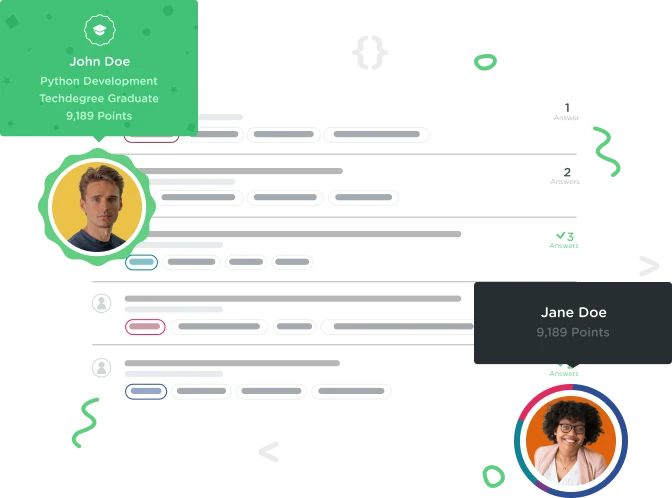
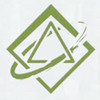
Jeremy Burghardt
4,037 PointsI am just not wrapping my head around this challenge, can someone walk me through this?
Alright, I honestly don't even know where to start here. I'm not sure why I'm not getting it, but it's just not clicking, and I'm at a loss.
Here is the challenge:
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
I make a function called arrayCounter, but what does it mean to take in a parameter which is an array? I know what an array is, and I know what the parameters are, but I'm not sure what it means to take in a parameter that's an array.
Honestly, if someone could just walk me through this, that'd be amazing. I thought I was understanding things fairly well, then all of a sudden I just don't know what the heck I need to do.
4 Answers

Ryan Duchene
Courses Plus Student 46,022 PointsNo problem man. Happens to all of us. Let's take this one step at a time:
First, it's asking us to make a function called arrayCounter()
.
function arrayCounter() {
// more code here later...
}
Then, it's telling us that this function that we just created, arrayCounter()
, takes a parameter (interchangeable with "argument," for future reference) that has to be an array.
function arrayCounter(arr) { // arr is the parameter that should be an array
}
Now, it's telling us to return the length of the array passed in (which, in our code, is arr
) and return it. Simple enough:
function arrayCounter(arr) {
return arr.length;
}
And, lastly, it's telling us to return 0
if the variable is a string, number, null, undefined, etc. To check if a variable is an array, just pass it into the Array.isArray()
method:
function arrayCounter(arr) {
if (Array.isArray(arr)) {
return arr.length;
} else {
return 0;
}
}
Did that help? If any of that was unclear, I'll go through it again. Happy coding!
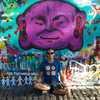
Andrew Stelmach
12,583 PointsI'm gonna take a stab at this and say that, for example: function arrayCounter(x) {whatever}; , 'x' is the parameter. You'd then call the function on another line with something like "blah(array);" where 'array' is a variable that is an array (you'd have assigned an array to this variable already). You have therefore created a function called 'arrayCounter' that has taken in a parameter that is an array.

Jason Anello
Courses Plus Student 94,610 PointsHi Jeremy,
The idea here is that to use this function you would pass an array into it and it will return the length of that array.
So this might be your array var ages = [37, 48, 95];
Then you would call the function like this arrayCounter (ages);
and that should return 3 because that's how many items there were.
Now, this function only counts items in an array. It's not for other data types.
What if you pass a number into this function by accident? arrayCounter(95)
You don't want to blindly assume that an array was passed in and then return the length of it.
So this challenge is all about checking the typeof
(hint hint) the variable that was passed in and only returning the length if it's an array. If it's one of the other 3 types mentioned then you should return 0.
The typeof
operator checks the type of a variable.
You only need to write the function here. You don't have to call it like I did above.
Let me know if you're still having trouble getting started.
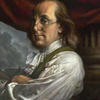
Jeff Busch
19,287 PointsHi Jeremy,
Hopefully this will help.
/*** Here we create a function called arrayCounter.
**** It takes an array as a parameter/argument.
**** This parameter happens to be named array. ***/
function arrayCounter(array) {
/*** Now we check the type or the array. If the array is of type
**** "string or || "number" or || "undefined" return 0. ***/
if (typeof array === "string" || typeof array === "number" || typeof array === "undefined") {
return 0;
/*** else, meaning if the array is not of any of those types,
**** return the length of the array. ***/
} else {
return array.length;
}
}
</script>
Of course, to do anything with this function it has to be called, for example: arrayCounter(arayPassedToFunction);
Jeff
Jeremy Burghardt
4,037 PointsJeremy Burghardt
4,037 PointsYes, this worked! Thanks, I appreciate the play-by-play style of explanation.
I have to say though, that challenge is a bit ridiculous. I don't recall ever learning that method, or if I did, it didn't stick with me. At the very least, I don't think it was recent enough for me to remember it for this challenge.
I also found an old post about this challenge here on Treehouse where people did this:
if (typeof arr === 'string' ||typeof arr === 'number' || typeof arr === 'undefined') { return 0; }
Which also worked. And which also drives me nuts, cause I don't recall ever learning about those double pipes either.
Anyhow, thanks for the help! This one was really getting to me :p
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsIn my answer I tried to give a solution that was doable based on what you've learned so far but this solution is the best way to go.
For one further improvement it would be better to return -1 if it's not an array but then it won't pass the challenge.
Ryan Duchene
Courses Plus Student 46,022 PointsRyan Duchene
Courses Plus Student 46,022 PointsNo problem! That's one of the reasons why the forum exists; we're here to help you if you ever run into problems like this.
Double pipes mean "or," by the way. And double ampersands (like
&&
) mean "and." And putting an exclamation point before a statement (so, like `if (!function(var))') will negate it. They're other things you'll run into while programming. :)Anyway, glad I could help!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI don't think that you learned the logical OR
||
operator either but that answer is given a lot too.If you're interested, I wrote a solution in this thread which I think is what you could reasonably come up with based on what you've learned so far.
https://teamtreehouse.com/forum/hi-could-you-please-tell-me-whats-wrong-with-this-answer
That solution does have problems though and Ryan's solution is the best way to go that I know of.