Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial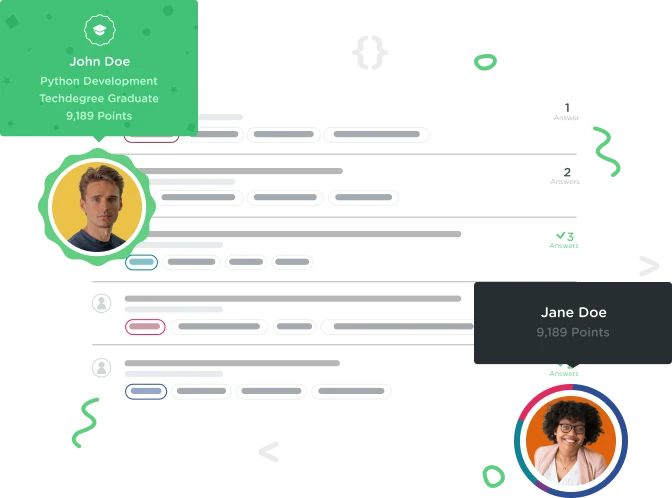

Mathew Yangang
4,433 PointsI am kind of confuse here
I am a little bit confuse here , not sure why my code is not passing
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, pattern):
result = []
fix_me = Letter(['_','.'])
for item in pattern:
if item =='dot':
result.append(".")
else:
result.append("_")
return "dash".join(result)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer

Duc Bui
14,546 Pointsclass Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, pattern):
pattern = pattern.split('-')
result = []
for item in pattern:
if item.lower() =='dot':
result.append(".")
elif item.lower() == 'dash':
result.append("_")
else:
pass
return cls(result)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
You're almost there, I only fix a few of your codes and it went through. You have to turn that string into a list first by using the split function and then you loop through that list and append "." if it is "dot" or "_" if it is "dash".
Mathew Yangang
4,433 PointsMathew Yangang
4,433 PointsGreat help .Thanks Duc Bui. But I do have a question though, why do you use the ("-") inside the split function? Can we just try to convert the string into a list without the ("-") inside the argument of .split(). Just curious...
Duc Bui
14,546 PointsDuc Bui
14,546 PointsMathew Yangang You have to indicate where to split the string
output = ['dash-dot']
output = ['dash', 'dot']
I think you do not understand how the split function works, check it out here: https://www.pythonforbeginners.com/dictionary/python-split