Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial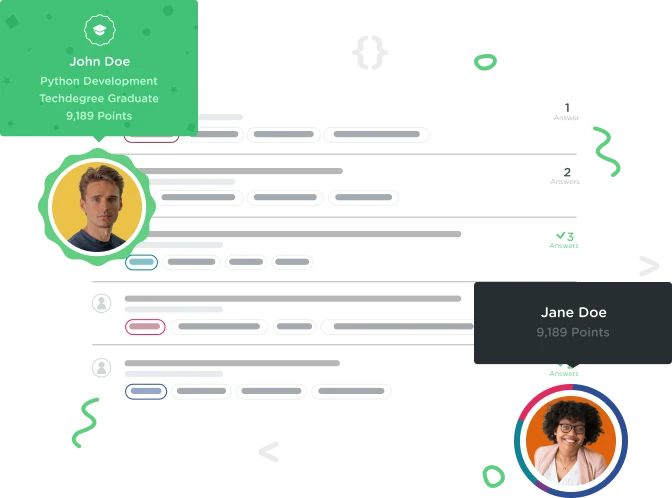

Christopher Bush
Courses Plus Student 8,382 PointsI am lost
I have watched the videos three times, and I am still coming up with nothing. Anybody have some good external resources I can look at to figure this stuff out?
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Objects</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Objects: Prototypes</h2>
<script>
var carPrototype = {
model: "generic",
currentGear: 0,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
function Car(model) {
}
</script>
</body>
</html>
3 Answers
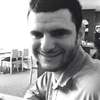
Dan Garrison
22,457 PointsI think I see what the issue is. The car prototype is first created with the following code:
var carPrototype = {
model: "generic",
currentGear: 0,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
Now you need to create a constructor function that can accept a value for model. This is logical in this situation because the model will be the value most likely to change.
This done using a constructor function, which would be created as follows:
function Car(model) {
this.model = model;
}
This will set value of the input to the model of the car. This completes the constructor function, but it is not yet complete. You next need to set this function to use the prototype. This would be done as follows:
Car.prototype = carPrototype;
Now you can call the constructor function with a value for model like this:
tesla = new Car("Tesla");
If you were call the variable tesla in the console now, you would see the model set to tesla and it would inherit all the properties of the car prototype.

shezazr
8,275 Pointssorry, I have used up all my psychic energy for today... please try letting us know what is wrong.

melvin van gent
8,536 PointsCan you be a little more specific about the error you get, and what you are trying to obtain? I can see a typo in your increaseGear method. Where the ++ is separated with a space from your local variable currentGear. I believe it also is good practice to use "+= 1" instead of "++" too.
A good source on prototypes in javascript can be found here: http://www.w3schools.com/js/js_object_prototypes.asp