Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial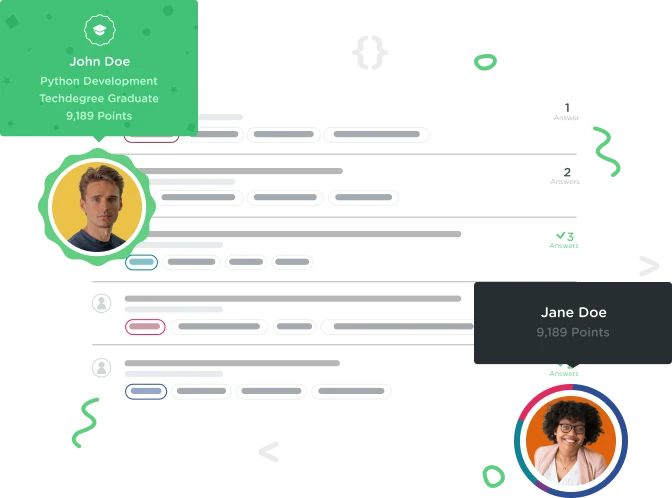
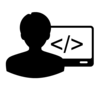
Abe Layee
8,378 PointsI am lost with instance and constructor object.
I am taking the JavaScript Object cousre and I have been doing good till I got to constructor object. Can anyone please explain to me what Instance and constructor objects are or what is instance in object? Thank you in advance. I copied these codes from the quiz. I have no idea how to identify which one is object or instance
function Monster(rank) { //constructor function
this.rank = rank;
this.health = 100;
this.takeHit = function() {
this.health--;
}
this.isDead = function() {
return this.health <= 0;
}
}
var monster = new Monster("Captain");
This is an instance which I copied from the challenge
function TodoListItem(description) {
this.description = description;
this.isDone = false;
this.toString = function() {
return this.description;
}
this.markAsComplete = function() {
this.isDone = true;
}
}
var task = new TodoListItem("Do the laundry!");
4 Answers
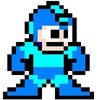
Robert Richey
Courses Plus Student 16,352 PointsHi Abraham,
Great question. First thing to get clear is that objects are new instances created by a constructor. Said another way, objects are instances, and they come from constructors. You can use object and instance interchangeably.
One way that helps me understand the difference is that a constructor is like a blueprint - it defines what a thing is. An object, is the actual thing built from the definition provided by a constructor.
Consider a house blueprint. That's a constructor that defines how to build a particular home. An actual house built using this blueprint would be a real object that someone could move into.
// constructor that creates new objects/instances
function House() {
var sqft = 1350;
var numFloors = 2;
var sizeOfFrontYard = 540;
var sizeOfBackYard = 720;
var exteriorColorScheme = { primary : 'gray', secondary : 'white' };
}
// house is a new object/instance created by the House constructor
var house = new House();
I hope this helps. Please let me know if you're still stuck and I'll figure out a way to help guide you closer to understanding this tricky concept.
Cheers!

Jeremy Fisk
7,768 PointsThe constructor function provides attributes and methods to an instance of an object that you create.
In your example, var monster
is an instance of the Monster
object, and the constructor function is a way of pre-defining what the Monster
object will consist of, upon instantiation through your code, :
var monster = new Monster("Captain");
so, since you defined that rank, health, and the two methods will be part of your Monster
object, anytime you create a Monster
from that point forward, it will consist of the attributes and functions present in your constructor function
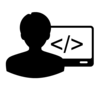
Abe Layee
8,378 Pointsaww thank, I think I undertand it now. I really appreciate your help Robert Richey .

Peter Furler
31,647 PointsTo Abraham,
To further clarify an instance is a single occurrence of something. In this case it is an object ( var house ) , built using the blue print of the constructor function ( House).
This enables you to create many different instances of the same type of object. All though each instance of the object will have the same properties and methods, they will more than likely have different values attached to their properties.
Abe Layee
8,378 PointsAbe Layee
8,378 PointsMy question is the last line of the code where you called the House() function or assign it to the House var. I know we use the new word to define a constructor object and your house() does not have. In this case, the House() is the instance and the last line of your code is the object? Right or not? I am sorry if you can't understand what I am trying to say here. I am just trying to wrap my head around it and thank again Robert Richey
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsNo worries, I know this is a confusing topic.
Instead of saying
var house = new House();
, we can call the new object/instance anything. Let's instead call itbacon
, because (almost) everyone loves bacon, and it doesn't matter what we call each new instance created by the constructor.