Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial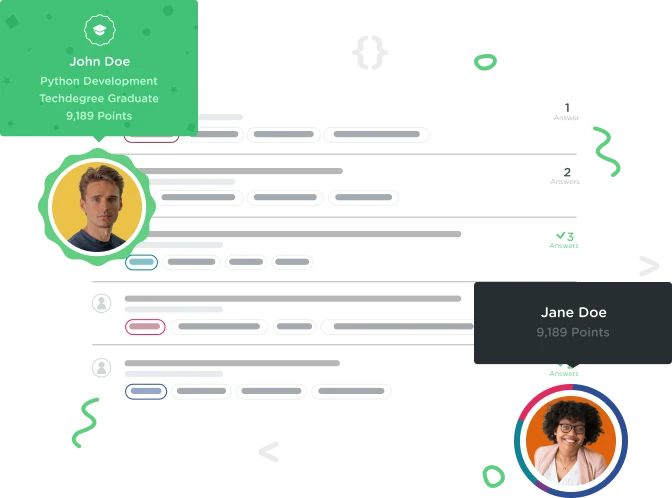

Sonam Vishwakarma
1,973 PointsI am not getting it please help me
I am not understanding it.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self, pattern):
s =""
for item in pattern:
if item == '.':
s += 'dot'
elif item == '_':
s += 'dash'
else:
s += item
return s
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer

ygh5254e69hy5h545uj56592yh5j94595682hy95
7,932 Points# We are creating a class called 'Letter
class Letter:
# we then create an __init__ which is the first thing that runs when you call the class Letter
# the __init__ has the following arguments; self, and pattern=None
# self refers to the class Letter
# pattern is variable set to None
def __init__(self, pattern=None):
# We have to define the variable pattern this the following code:
self.pattern = pattern
# __str__ is a method that is used to represent string of the object
# we give the arguments, self and pattern to it
# again, self just refers to the class Letter
# and pattern refers to the pattern that is assign in __init__ method
def __str__(self, pattern):
# creating a variable called 's' with an empty string
# this will hold our morse code
s =""
# we then look through all the pattern
# for example, pattern = ['.', '.', '.'], pattern is an array
# that holds multiple elements. with for item in pattern we
# are going to look through each element and do the following:
for item in pattern:
# if (item) 'element' is a '.'(period), we add it as 'dot' in s (variable)
if item == '.':
s += 'dot'
# if (item) 'element' is a '_'(underscore), we add it as 'dash' in s (variable)
elif item == '_':
s += 'dash'
# else, just add what ever item is to s (variable)
else:
s += item
# then we are just returning the variable s
return s
# Here we create a child class S of Letter
# meaning that Letter is parent of S
# the reason why we create a child class is
# because of inheritance
class S(Letter):
# again __init___ is the method that will run first when you call child S Class
# __init__ is not referring to Letter's __init__, they are their own methods, for now
def __init__(self):
# here we create the 'Morse code' pattern
pattern = ['.', '.', '.']
#super() allows us to call the parent Letter and give it the pattern array
#that would later be converted into the desired pattern, ex.: 'dashdashdash'/'dotdashdot'
super().__init__(pattern)