Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial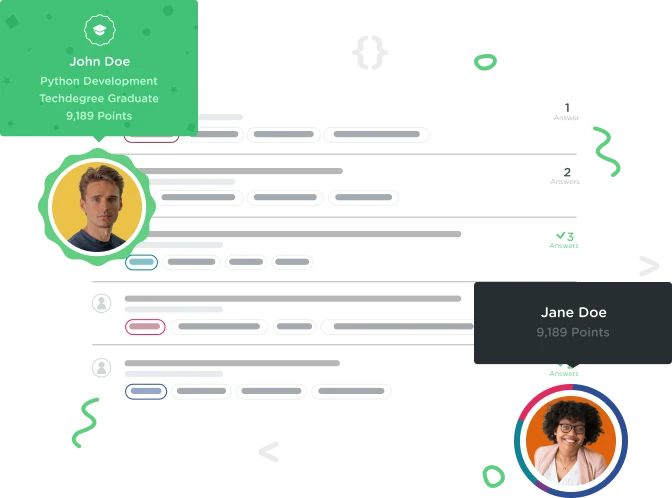

Aniket Kumar
3,266 PointsI am not sure how I am supposed to use closures with a function as opposed to constant or a variable.
The task: We're writing an app to fetch the most recent blog posts from the Treehouse blog. This requires making a network request using asynchronous methods that execute in the background. For that we need a closure. Create a method called fetchTreehouseBlogPosts, that has a single parameter - a completion handler. The closure has three parameters: a data object containing the results of the request as type NSData!, the HTTP response object from our request as type NSURLResponse!, an error object as type NSError!, and a return type of void. Note: If you prefer to use a typealias to make the method signature clear, name it BlogPostCompletion
import Foundation
// Add your code below
func fetchTreehouseBlogPosts(completion: ([String:AnyObject]?) -> Void) {
let data = NSData!
let response = NSURLResponse!
let error = NSError!
return ()
}
1 Answer

Matthew Young
5,133 PointsAll we're doing here is just setting up the function declaration and its parameters. To create a closure as the parameter, you're pretty much doing the same as declaring a variable or constant. First, you name the parameter and then declare its type. The name in this case can be anything, but since we're creating a completion closure, I would just call it "completion". Then you need to declare the type. The type is going to look similar to another function declaration, however you're not going to define the name of the parameters. The completion handler is going to contain NSData, NSURLResponse, and NSError. There is nothing that's returned so the return type is Void. All that's left is just putting it all together.
func fetchTreehouseBlogPosts(completion: ((NSData!, NSURLResponse!, NSError!) -> Void)) { }
A typealias can be used to make the code more legible. You can do so by doing this:
typealias BlogPostCompletion = ((NSData!, NSURLResponse!, NSError!) -> Void)
func fetchTreehouseBlogPosts(completion: BlogPostCompletion) { }
Personally, I feel that closures are a bit harder to grasp than most other concepts of Swift. I'm still trying to understand them better myself. I would just read up on them in the Swift Programming Language guide that Apple provides. It helps tremendously to better understand closures. Here's a link to it: Swift Programming Language: Closures
I hope my explanation helped!