Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial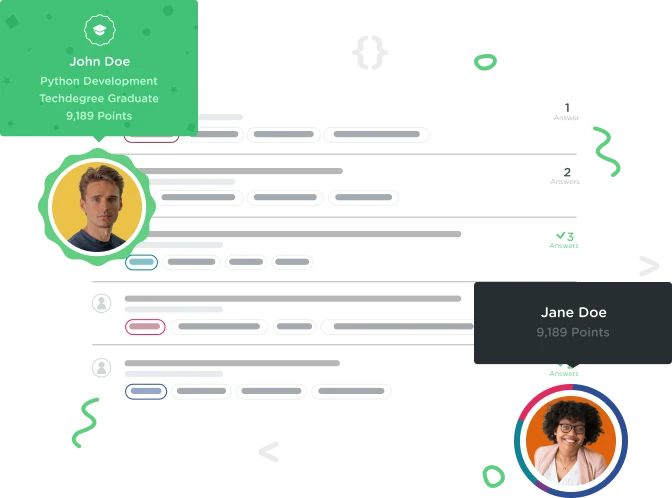

Caroline Gould
1,467 PointsI am not sure how to make this work without an if statement. Don't we need to evaluate "if" the tile is present or not?
The question prompts us to index into the string, which I am doing. The source code has a boolean in it. Are we meant to delete it? I am not sure how to use the boolean without an "if" statement.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
boolean tileThere = tiles.indexOf(tile)!=-1;
if(tileThere)
{
return true;
}
return tileThere;
}
}
4 Answers
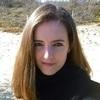
Linda de Haan
12,413 PointsYou could do it like this:
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
This method returns a boolean and uses the indexOf method, which you already got to check whether or not the tile is in the tiles string. You don't have to store it first, you can just return it like this.
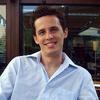
Pedro Cabral
33,586 PointsPerhaps you should return the expression from the boolean straight away instead of storing it?

Caroline Gould
1,467 Pointsgot it :)
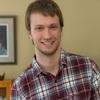
Patrick Bluth
13,285 PointsHey Caroline,
Your answer is really close and pretty much right. However, you don't even need to store the boolean like you did at all. Instead, you can simply return that line like so:
public boolean hasTile(char tile) {
return (tiles.indexOf(tile) != -1);
}
This is saying that when hasTile is called, return true if the letter is in the Tiles string (ie the index is NOT equal to -1). If the letter is not in the Tiles string (ie the index is equal to -1), then this return statement is equal to false, and false will be returned.