Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial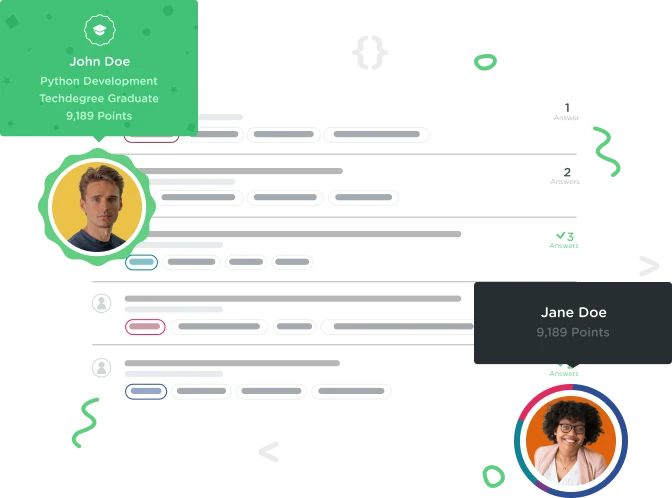

Noah Wasserman
955 PointsI am not sure how to solve this, can Someone walk me through this step by step
The prompt:
Alright, this one can be tricky but I'm sure you can do it.
Create a function named combo that takes two ordered iterables. These could be tuples, lists, strings, whatever.
Your function should return a list of tuples. Each tuple should hold the first item in each iterable, then the second set, then the third, and so on. Assume the iterables will be the same length.
Check the code below for an example.
I cannot figure out what to do, I can easily just find the answers online but I still don't understand why they do what they did. Can someone please take the time and explain to me step by step what, why, and how. I would very much appreciate it
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo (iterable1, iterable2):
for index, x in enumerate(iterable1):
2 Answers

Cooper Runstein
11,850 PointsI'm sure there are a million way to solve this, but here's what I chose to do.
def combo(iterable1, iterable2):
tuples_list =[] #Create an empty list to store the tuples we'll create in.
#Assuming equal lengths, we're going to create an index for each element in both iterables
for idx in range(0, len(iterable1)):
#using the indexs we created, we can access that index on both iterables, and append it to the tuples_list. this could also be done in two parts, creating a variable named 'tuple' and then appending that, that's your choice.
tuples_list.append((iterable1[idx], iterable2[idx]))
return tuples_list #return our filled in list
As you're clearly curious enough to actually want to know how this works, I'd recommend when you finish this course ou look into the more pythonic method 'zip'
#this won't pass the challenge, but it's the way you'd normally solve this
def combo(iterable1, iterable2):
return list(zip(iterable1, iterable2))
Let me know if you need any more clarifications

Noah Wasserman
955 Pointswhy not use the enumerate instead of range?

Cooper Runstein
11,850 Pointsdef combo (iterable1, iterable2):
tuples =[]
for index, x in enumerate(iterable1):
tuples.append((iterable1[index], iterable2[index]))
return tuples
Like above? You could, for this example, there's no particular reason to use one over the other. I like using range because it seems clearer as to what's going on, but that's entirely my opinion. They both work, and they both are very similar in how they work. I believe that range is a slightly faster option, but it's a micro improvement, unless you're calling the function thousands of times in a row, there isn't much of an advantage to either.
Bryan Land
10,306 PointsBryan Land
10,306 PointsYou cannot append() with more than one argument...
Cooper Runstein
11,850 PointsCooper Runstein
11,850 PointsBrayn, yes, that's correct. I see I left out the () as I meant to append a tuple, not two arguments. I'll change that.