Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial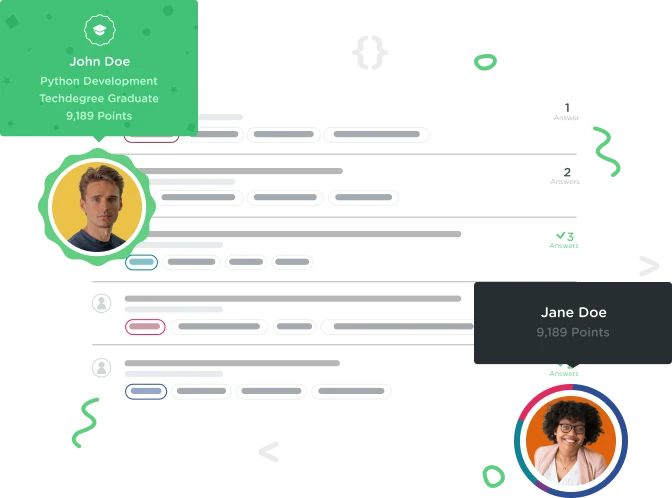

Jordon Crichton
1,868 PointsI am not sure what I am missing. The error says I am not hurting when hitting the wall.
Our move function takes this three-part tuple player and a direction tuple that's two parts, the x to move and the y (like (-1, 0) would move to the left but not up or down).
Finish the function so that if the player is being run into a wall, their hp is reduced by 5. Don't let them go past the wall. Consider the grid to be 0-9 in both directions. Don't worry about keeping their hp above 0 either.
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
if direction == (-1,0):
x-=1
elif direction == (1,0):
x+=1
elif direction == (0,1):
y+=1
elif direction == (0,-1):
y-=1
elif direction == (-1,0) and x == 0:
x = 0
hp -= 5
elif direction == ( 1,0) and x == 9:
x = 9
hp -= 5
elif direction == (0,-1) and y == 0:
y=0
hp -= 5
elif direction == (0,1) and y == 9:
y=9
hp -=5
return x, y, hp
1 Answer
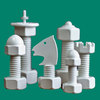
Steven Parker
230,274 PointsRemember that an "if/elif" chain is efficient because it stops testing when any condition is met. And the first few tests look only at direction, so if they match, they will change a coordinate and not change the hp, even if a wall is being hit.
A few things to keep in mind while implementing this function:
- don't assume the movement will be in only one direction
- don't assume the motion will be only one step
- check for wall collisions first
- if a wall would be hit, change the hp but not the location