Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial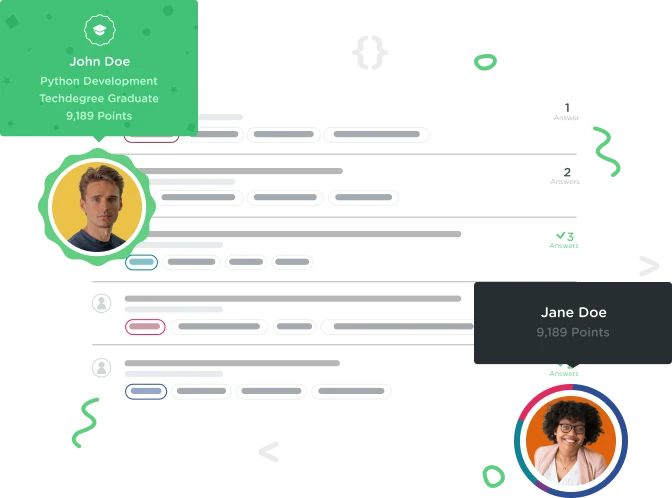

josh hahn
5,926 PointsI am not sure what is being asked for here. I am not sure what he means by get a handle on
nothing further
package com.example;
import com.example.model.Brand;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
public class Controller {
@FXML
private VBox container;
public void handlePlay(ActionEvent actionEvent) {
System.out.println("[MUSIC PLAYING] - I shot the sheriff, but I didn't shoot the deputy");
}
public void switchBrand(Brand brand) {
// Help me clear the old, and add the proper styles
clearBrandStyles();
}
public void clearBrandStyles() {
for (Brand brand : Brand.values()) {
container.getStyleClass().remove(brand.toString().toLowerCase());
}
}
public void handleBrandSwitch(ActionEvent actionEvent) {
// Get a handle on the calling button
Button btn = (Button) actionEvent.getSource();
// Get access to the brand like Brand.APPLE by name
Brand brand = Brand.valueOf(btn.getText().toUpperCase());
switchBrand(brand);
}
}
/* Brand settings */
.sony {
-fx-background-color: black;
}
.jvc {
-fx-background-color: red;
}
.apple {
-fx-background-color: silver;
}
.coby {
-fx-background-color: gray;
}
package com.example;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("boombox.fxml"));
primaryStage.setTitle("Boombox");
primaryStage.setScene(new Scene(root, 300, 275));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
<?import javafx.scene.control.Button?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.layout.VBox?>
<?import javafx.scene.text.Text?>
<VBox fx:controller="com.example.Controller"
xmlns:fx="http://javafx.com/fxml"
stylesheets="@boombox.css"
fx:id="container">
<HBox>
<Button onAction="#handleBrandSwitch">Sony</Button>
<Button onAction="#handleBrandSwitch">Apple</Button>
<Button onAction="#handleBrandSwitch">JVC</Button>
<Button onAction="#handleBrandSwitch">Coby</Button>
</HBox>
<Text text="Boombox" />
<Button onAction="#handlePlay" text="Play"/>
</VBox>
package com.example.model;
public enum Brand {
JVC("JVC"),
SONY("Sony"),
APPLE("Apple"),
COBY("Coby");
private String mDisplayName;
Brand(String displayName) {
mDisplayName = displayName;
}
public String getDisplayName() {
return mDisplayName;
}
}
1 Answer
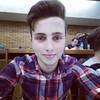
Rares Conea
Courses Plus Student 15,000 PointsHi,
You are required to write code only in switchBrand(), you don t care about the other methods. First you must clear the current brands in order to add a new one and you did this by calling clearBrandStyle(); After that you must add the one passed as parameter to your method(in the same method after clearBrandStyle()): container.getStyleClass().add(brand.toString().toLowerCase());