Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial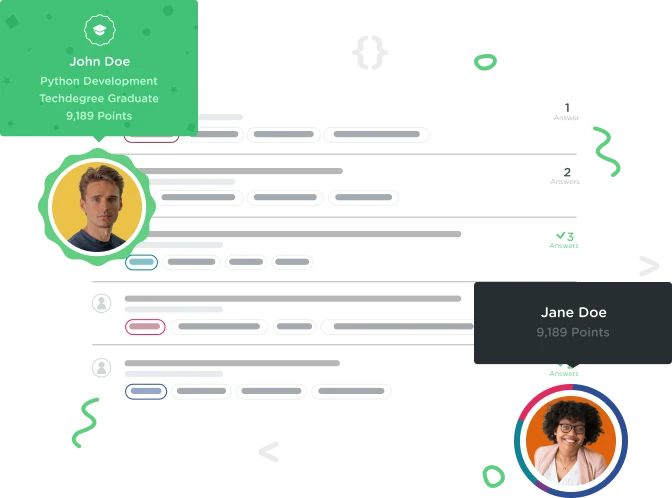
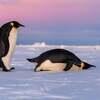
fionaj
1,380 PointsI am not sure what this code challenge wants me to do.
I am confused on how to return a string that says 'Bao Bao eats bamboo.' where 'Bao Bao' is the name attribute and 'bamboo' is the food attribute.
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
is_hungry = False
print("Bao Bao eats bamboo.")
2 Answers
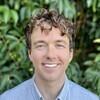
Asher Orr
Python Development Techdegree Graduate 9,410 PointsHi Fiona!
When you click "Check Work," the Treehouse checker makes an instance of your Panda class. It runs code like this:
treehouse_checkers_instance = Panda('Bao Bao', '17')
Here's how it parses your code:
class Panda:
species = 'Ailuropoda melanoleuca'
#ok, the species attribute of ANY instance of the Panda class is 'Ailuropoda melanoleuca'
#self.species will always be 'Ailuropoda melanoleuca'
food = 'bamboo'
#ok, the food attribute of ANY instance of the Panda class will be 'bamboo'.
#self.food will always be 'bamboo.'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
#when treehouse_checkers_instance was created, I set the name attribute to 'Bao Bao'
#for this instance, self.name is 'Bao Bao'.
self.age = age
#when treehouse_checkers_instance was created, I set the age attribute to '17'
#for this instance, self.name is '17'.
Then it calls the eat method. Like this:
treehouse_checkers_instance = Panda('Bao Bao', '17')
treehouse_checkers_instance.eat()
The eat method should change the is_hungry attribute to False. It should also return the string 'Bao Bao eats bamboo.'
Look at line 2 in your eat method:
def eat(self):
is_hungry = False
print("Bao Bao eats bamboo.")
All instance attributes need the "self." prefix.
self.is_hungry = False
#the line above actually means:
treehouse_checkers_instance.is_hungry = False
Here's how to fix the last line:
- Make sure you return the string (rather than printing it.)
- Format the string to hold the name and food attributes.
I hope this helps. Let me know if you have questions!
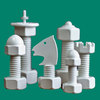
Steven Parker
241,809 PointsThe string 'Bao Bao eats bamboo.' is simply an example to show the expected output formatting. The challenge wants you to construct the message using the name attribute that was stored during instance creation (which would most likely be something other than 'Bao Bao') and the food attribute in the class definition.
Also note that the instructions say to return the string instead of printing it.
Finally, remember that when referring to class or instance attributes, use the prefix self.
.
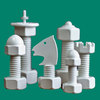
Steven Parker
241,809 PointsHi again, fionaj,
The food attribute is already part of the class, defined on this line:
food = 'bamboo'
So you don't need to create it, just refer to it when you are building the formatted string. An example of using the name attribute in a string might look like this:
print(f"{self.name} is my name!")
Just remember that for this challenge you will be returning the string and not printing it, and you need to use both the name and food attributes to make a string that looks like the example.
fionaj
1,380 Pointsfionaj
1,380 PointsHello thanks for help me out. I understand what to do now, but I am unsure what "Also, return a string that says 'Bao Bao eats bamboo.' where 'Bao Bao' is the name attribute and 'bamboo' is the food attribute." is supposed to mean. Am i supposed to create a food attribute?