Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial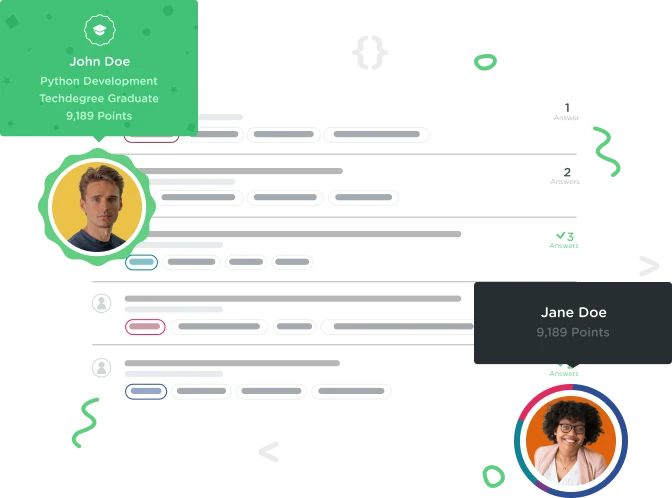
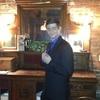
Carl Smith
8,185 PointsI am not understanding how to do this task.
I'm just not understanding the instructions. Not sure where to begin....
import Foundation
enum UIBarButtonStyle {
case Done
case Plain
case Bordered
}
class UIBarButtonItem {
var title: String?
let style: UIBarButtonStyle
var target: AnyObject?
var action: Selector
init(title: String?, style: UIBarButtonStyle, target: AnyObject?, action: Selector) {
self.title = title
self.style = style
self.target = target
self.action = action
}
}
enum Button {
case Done(String)
case Edit(String)
}
let done = Button.Done("Done")
1 Answer
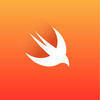
Steven Deutsch
21,046 PointsHey Carl Smith,
You need to create an instance method called toUIBarButtonItem(). The method will be associated with the Button class, because it is called on an instance of Button. This method has to return a UIBarButtonItem.
What you want to do is create a switch statement inside of this method (function), and switch on self. In this case, self is referring to the specific instance. Remember, this is an instance method so it will be called on an instance of the Button type. Here, self refers to whatever instance it is being called on.
You then want to create case statements to match for all the Button enum's members. This would be Done and Edit.
For each of those cases, you need to return an instance of UIBarButtonItem, using its initializer method. This initializer takes 4 arguments. For target and action you can pass in nil. For the style you have to use .Done for the Done case and .Plain for the Edit case. These arguments are specified in the directions.
For the title, you want to extract the associated value from the instance you are switching on. You can do this by assigning it to a constant. You can then pass that constant in for the title argument.
Now you have your instance method set up, you just need to call it on the instance of Button you created earlier (done) and assign the returned UIBarButtonItem to a constant called doneButton.
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case Done(let title):
return UIBarButtonItem(title: title, style: .Done, target: nil, action: nil)
case Edit(let title):
return UIBarButtonItem(title: title, style: .Plain, target: nil, action: nil)
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem()
Good Luck!