Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial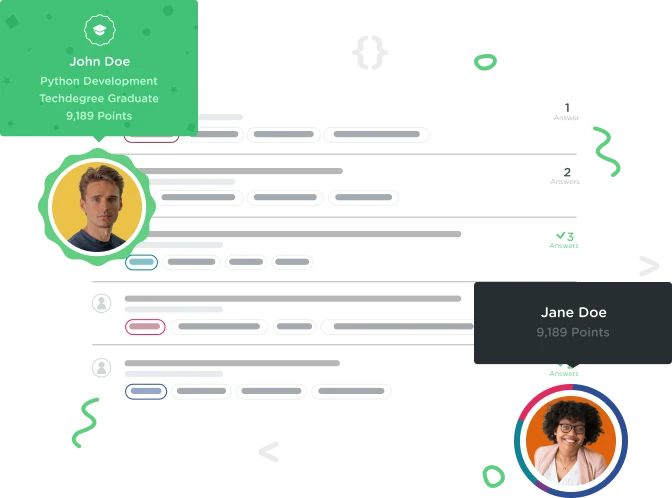

I-Chun Chen
1,712 PointsI am not understanding~ need help!!
I am confused with the task!
import Foundation
enum UIBarButtonStyle {
case Done(String)
case Plain
case Bordered
}
class UIBarButtonItem {
var title: String?
let style: UIBarButtonStyle
var target: AnyObject?
var action: Selector
init(title: String?, style: UIBarButtonStyle, target: AnyObject?, action: Selector) {
self.title = title
self.style = style
self.target = target
self.action = action
}
}
let done = UIBarButtonItemStyle.Done("Done")
enum Button {
case Done(String)
case Edit(String)
}
5 Answers
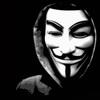
Gabriel Mititelu
5,881 PointsHello, I-Chun Chen.
The first part of the code challenge is asking you to assign an enum value of type Button with the member Done to a constant name done, and to assign the string "Done" as the associated value. Like this:
let done: Button = .Done("Done")
The second part asks you the following:
"To the button enum, add a method named toUIBarButtonItem that returns an instance of UIBarButtonItem configured properly".
Pretty straightforward, this should look somewhat like this:
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
}
}
"In the buttons.swift file there is a basic implementation of UIBarButtonItem. You can create buttons with three different styles and titles."
"Using the associated values as titles for the button, return a button with style UIBarButtonStyle.Done for the Done member of the Button enum. Similarly for the Edit member, return a UIBarButtonItem instance with the style set to UIBarButtonStyle.Plain. In both cases you can pass nil for target and action. "
Okay. So this is what I've got to do, but how do I do it? Well, I recommend using a switch statement as it's the best choice for the task at hand; for each member of the Button enum , we want a UIBarButtonItem returned. This should look somewhat like this:
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done: return UIBarButtonItem(title: "Done", style: .Done, target: nil, action: nil)
case .Edit: return UIBarButtonItem(title: "Edit", style: .Plain, target:nil, action: nil)
}
}
}
" Once you have a method, call it on the value we created in the previous task and assign it to a constant named doneButton."
Okay. This is also pretty straightforward, so this should look like this:
let doneButton = done.toUIBarButtonItem()
This should get you through the challenge. :)
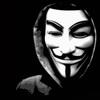
Gabriel Mititelu
5,881 PointsNormally, your code should compile. However, as the environment is sort of limited, I suppose it expects you to declare the type explicitly when declaring the constant.
let done: Button
Since you're declaring the type explicitly, the constant knows the type and you could access the enum cases using only " .case" instead of "Button.case". This shouldn't be a requirement, however , as both cases are equally correct -- one's a shortcut.
Give it a go with the type declared explicitly. If that alone doesn't do it, try using the shortcut too. :)

Carmen Ibarcena
iOS Development Techdegree Student 8,296 PointsHello Gabriel Mititelu,
I have a question for you I am having the same problem with this challenge this is my code for the first task:
enum Button { case Done(String) }
let done: Button = .Done("Done")
but i still have an error this is my error " Bummer! Make sure you're assigning the enum value to a constant named done can you please help me! Thank you so much
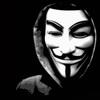
Gabriel Mititelu
5,881 PointsHi Carmen,
You ought to be in the same file with the Button enum and make sure you're assigning it outside of the enum ( after the closing curly bracket.

Carmen Ibarcena
iOS Development Techdegree Student 8,296 PointsYes I figure out Thank you so much =)
I-Chun Chen
1,712 PointsI-Chun Chen
1,712 PointsFirst, thanks for answering my question :) but I still confused with the task 1 of 2.The task is to assign an enum value of type Button with the member Done. so there is my code:
enum Button { case Done(String)}
let done = Button.Done("Done")
But it still can’t work. It said “Make sure you're assigning the enum value to a constant named done.” I tried the different ways to find out the answer out,but I am not good enough to solve it. Could you help me to figure out the problem? Again, thanks for your explicit answer.I really appreciate :)