Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial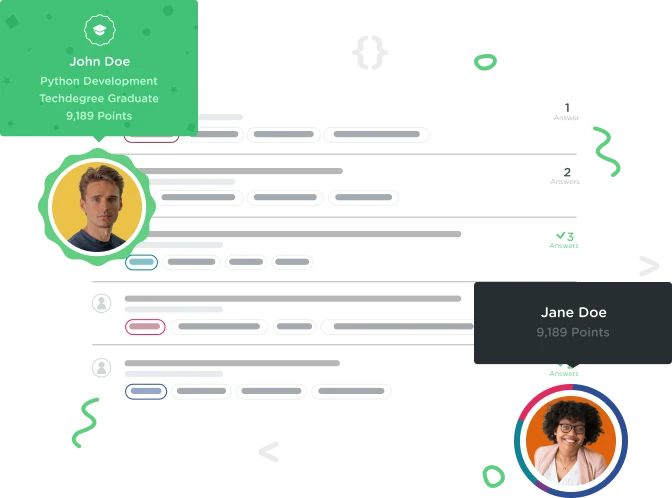
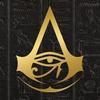
Jack Ripper
3,792 PointsI am only getting a bunch of zeros I dont know where I went wrong
package com.example.pc.stormy;
import java.sql.Time;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
import java.util.logging.SimpleFormatter;
public class CurrentWeather {
private String locationLabel;
private String icon;
private long time;
private double temperature;
private double humidity;
private double precipChance;
private String summary;
private String timeZone;
public String getTimeZone() {
return timeZone;
}
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
public String getLocationLabel() {
return locationLabel;
}
public void setLocationLabel(String locationLabel) {
this.locationLabel = locationLabel;
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public CurrentWeather() {
}
public CurrentWeather(String locationLabel, String icon, long time, double temperature,
double humidity, double precipChance, String summary, String timeZone) {
this.locationLabel = locationLabel;
this.icon = icon;
this.time = time;
this.temperature = temperature;
this.humidity = humidity;
this.precipChance = precipChance;
this.summary = summary;
this.timeZone = timeZone;
}
public int getIconId(){
int iconId = R.drawable.clear_day;
switch (icon){
case "clear-day":
iconId = R.drawable.clear_day;
break;
case "clear-night":
iconId = R.drawable.clear_night;
break;
case "rain":
iconId = R.drawable.rain;
break;
case "snow":
iconId = R.drawable.snow;
break;
case "sleet":
iconId = R.drawable.sleet;
break;
case "wind":
iconId = R.drawable.wind;
break;
case "fog":
iconId = R.drawable.fog;
break;
case "cloudy":
iconId = R.drawable.cloudy;
break;
case "partly-cloudy-day":
iconId = R.drawable.partly_cloudy;
break;
case "partly-cloudy-night":
iconId = R.drawable.cloudy_night;
break;
}
return iconId;
}
public long getTime() {
return time;
}
public String getFormattedTime() {
SimpleDateFormat formatter = new SimpleDateFormat("h:mm a");
formatter.setTimeZone(TimeZone.getTimeZone(timeZone));
Date dateTime = new Date(time * 1000);
return formatter.format(dateTime);
}
public void setTime(long time) {
this.time = time;
}
public double getTemperature() {
return temperature;
}
public void setTemperature(double temperature) {
this.temperature = temperature;
}
public double getHumidity() {
return humidity;
}
public void setHumidity(double humidity) {
this.humidity = humidity;
}
public double getPrecipChance() {
return precipChance;
}
public void setPrecipChance(double precipChance) {
this.precipChance = precipChance;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
}
package com.example.pc.stormy;
import android.content.Context;
import android.databinding.DataBindingUtil;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.nfc.Tag;
import android.support.v4.net.ConnectivityManagerCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.text.method.LinkMovementMethod;
import android.util.Log;
import android.widget.TextView;
import android.widget.Toast;
import com.example.pc.stormy.databinding.ActivityMainBinding;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather currentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
final ActivityMainBinding binding = DataBindingUtil.setContentView(MainActivity.this,
R.layout.activity_main);
TextView darkSky = findViewById(R.id.darkSkyAttribution);
darkSky.setMovementMethod(LinkMovementMethod.getInstance());
String apiKey = "HIDDEN BY MOD";
double latitude = 37.8267;
double longitude = -122.4233;
String forecastURL = "https://api.darksky.net/forecast/"
+ apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastURL)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
currentWeather = getCurrentDetails(jsonData);
CurrentWeather dsplayWeather = new CurrentWeather(
currentWeather.getLocationLabel(),
currentWeather.getIcon(),
currentWeather.getTime(),
currentWeather.getTemperature(),
currentWeather.getHumidity(),
currentWeather.getPrecipChance(),
currentWeather.getSummary(),
currentWeather.getTimeZone()
);
binding.setWeather(dsplayWeather);
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "IO Exception caught: ", e);
} catch (JSONException e) {
Log.e(TAG,"JSON Exception caught");
}
}
});
}
Log.d(TAG,"Main UI code is running, horay!");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON" + timezone);
JSONObject currently = forecast.getJSONObject("currenty");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setLocationLabel("Europe/Tirane");
currentWeather.setPrecipChance(currently.getDouble("precipPropability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}else {
Toast.makeText(this, getString(R.string.network_unavailable_message), Toast.LENGTH_LONG).show();
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
<variable
name="weather"
type="com.example.pc.stormy.CurrentWeather"/>
</data>
<android.support.constraint.ConstraintLayout
android:id="@+id/iconImageView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/appBackground"
tools:context=".MainActivity">
<TextView
android:id="@+id/temperatureValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="@{String.valueOf(Math.round(weather.temperature)), default=`100`}"
android:textColor="@android:color/white"
android:textSize="150sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/degreeImageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
app:layout_constraintStart_toEndOf="@+id/temperatureValue"
app:layout_constraintTop_toTopOf="@+id/temperatureValue"
app:srcCompat="@drawable/degree" />
<TextView
android:id="@+id/timeValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="@{`At ` + String.valueOf(weather.formattedTime) + ` it will be`, default=`At 5:00 PM it will be`}"
android:textColor="@color/half_white"
android:textSize="18sp"
app:layout_constraintBottom_toTopOf="@+id/temperatureValue"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
<TextView
android:id="@+id/locationValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="24dp"
android:text="Kukes Albania"
android:textColor="@android:color/white"
android:textSize="24sp"
app:layout_constraintBottom_toTopOf="@+id/timeValue"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="64dp"
app:layout_constraintBottom_toBottomOf="@+id/locationValue"
app:layout_constraintStart_toStartOf="parent"
app:srcCompat="@drawable/cloudy_night" />
<android.support.constraint.Guideline
android:id="@+id/guideline"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.33" />
<android.support.constraint.Guideline
android:id="@+id/guideline2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintGuide_percent="0.66" />
<TextView
android:id="@+id/humidityLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:paddingTop="15dp"
android:text="humidity"
android:textColor="@color/half_white"
app:layout_constraintEnd_toStartOf="@+id/guideline"
app:layout_constraintStart_toStartOf="@+id/guideline"
app:layout_constraintTop_toBottomOf="@+id/temperatureValue" />
<TextView
android:id="@+id/humidityValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingTop="15dp"
android:text="@{String.valueOf(weather.humidity), default=`0.88`}"
android:textColor="@android:color/white"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="@+id/humidityLabel"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="@+id/humidityLabel"
app:layout_constraintTop_toBottomOf="@+id/humidityLabel" />
<TextView
android:id="@+id/precipLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:paddingTop="15dp"
android:text="Rain Snow?"
android:textColor="@color/half_white"
app:layout_constraintEnd_toStartOf="@+id/guideline2"
app:layout_constraintStart_toStartOf="@+id/guideline2"
app:layout_constraintTop_toBottomOf="@+id/temperatureValue" />
<TextView
android:id="@+id/precipValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingTop="15dp"
android:text="@{String.valueOf(Math.round(weather.precipChance * 100)) + ` %`, default=`50 %`}"
android:textColor="@android:color/white"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="@+id/precipLabel"
app:layout_constraintHorizontal_bias="0.629"
app:layout_constraintStart_toStartOf="@+id/precipLabel"
app:layout_constraintTop_toBottomOf="@+id/precipLabel" />
<TextView
android:id="@+id/summaryValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:text="@{weather.summary, default=`Stormy with a chance of meatballs`}"
android:textColor="@android:color/white"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/precipValue" />
<TextView
android:id="@+id/darkSkyAttribution"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginEnd="8dp"
android:layout_marginBottom="8dp"
android:text="@string/dark_sky_message"
android:textColor="@color/half_white"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>
</layout>
1 Answer

Lauren Moineau
9,483 PointsHi. Are you talking about zeros on the UI? So the temperature, humidity and rain/snow all display zero? Are you getting any error/message in your logcat?
A small piece of advice: do not share your API key publicly. Leave a blank String with a comment.
NB. To fully format your class or layout file, surround it with 3 backticks (```) and add java in parentheses at the top for syntax highlighting.
(3 backticks)(java)
// your pasted code
(3 backticks)