Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial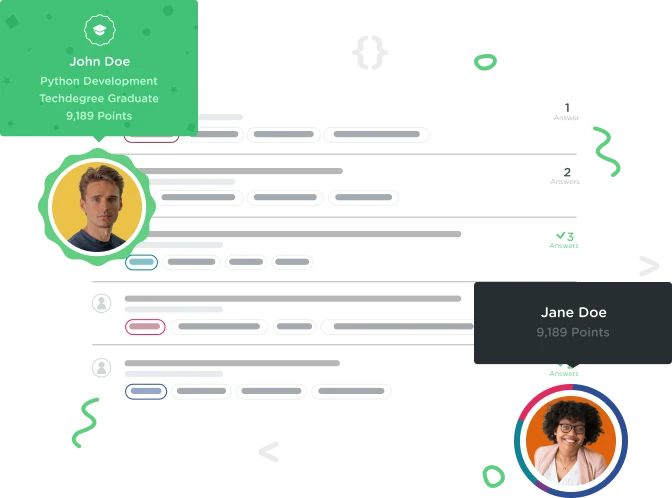

Joshua Faisal
4,493 PointsI am really confused how to know if product_idea is less than 3 characters. Can someone please explain it to me?
def suggest(product_idea):
return product_idea + "inator"
if len(product_idea) < 3:
raise ValueError
1 Answer
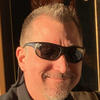
Peter Vann
36,425 PointsHi Joshua!
Your if statement is the correct test to see if the product_idea string is long enough (or not).
Your logic (order of operation) is out-of-proper-sequence, though.
You want to first test the string length.
Then, if it's too short, raise the exception.
But, if it isn't (think: else), then concatenate "inator" to it and return it.
Like this:
def suggest(product_idea):
if len(product_idea) < 3:
raise ValueError("Name must be a least 3 characters long.")
else:
return product_idea + "inator"
That passed.
I also tested it here:
https://www.katacoda.com/courses/python/playground
Using this code:
def suggest(product_idea):
if len(product_idea) < 3:
raise ValueError("Name must be a least 3 characters long.")
else:
return product_idea + "inator"
print( suggest("Term")) # pass
print("") # blank line
print( suggest("TM")) # fail
Ran it like this:
root@e6d2d7627308:/root# python3 app.py
And got this (expected/correct result):
Terminator
Traceback (most recent call last):
File "app.py", line 11, in <module>
print( suggest("TM")) # fail
File "app.py", line 3, in suggest
raise ValueError("Name must be a least 3 characters long.")
ValueError: Name must be a least 3 characters long.
I hope that helps.
Stay safe and happy coding!
Joshua Faisal
4,493 PointsJoshua Faisal
4,493 PointsThanks!