Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial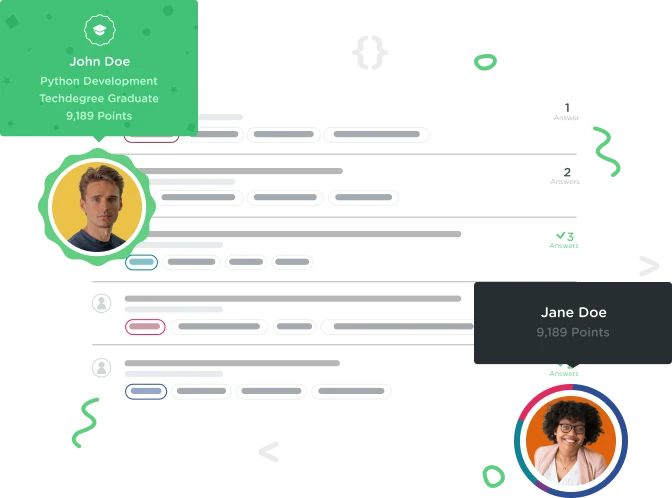

Hannah Elliott
11,001 PointsI am so lost. I've rewatched the entire Functions set of videos.
help!

elk6
22,916 Points@Kris, he keeps on posting it. I just deleted another copy.
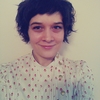
Kristopher Van Sant
Courses Plus Student 18,830 PointsThanks Elian, I've removed several from other posts as well.
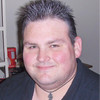
Roy Penrod
19,810 PointsHey, guys ... Krondor just keeps posting that crap everywhere. Here's another thread where he posted it:
https://teamtreehouse.com/community/help-375
If you look at his profile, he's using Nick Pettit's photo and none of the links to his social media profiles are for his. It looks like he's just a troublemaker.
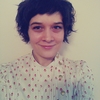
Kristopher Van Sant
Courses Plus Student 18,830 PointsThanks Roy! Just removed that one and sent an email to the Community manager.

elk6
22,916 Points@Roy: Yeah, i saw that he has Nick's profile pic. Bunch of nonsense posted there as well. Was just about to send the CM a message but i see Kris already did it. :)
Might send a message anyway.
2 Answers

elk6
22,916 PointsHi Hannah,
I will break the challenge down in segments.
First, the challenge asks you to make a function, making a function always start with the keywork "function". This way, the javascript interpreter knows you are making a new function. Next, the challenge asks you to name the function "returnValue". In this case, you would write the name exactly after the function keyword. So, so far we have:
function returnValue
Next, the challenge asks you to give the function a parameter. These are optional, but when provided you can use them later in the function, do something with it if you will. The argument always comes in parentheses after the function name, if you provide one. A function does not necessarily need an argument but for the challenge we need one. I will show in more depth what we do with the argument in breaking down task 2. So, so far, we have:
function returnValue(argument)
I have named the argument simply "argument" in the example but you can name it anything you want.
Next, we open the function with a pair of brackets, this creates the body of the function. Inside, you can declare what you want the function to do. So:
function returnValue(argument {
}
Lastly, for task one, the challenge wants to return the argument you passed to the function immediately. So, inside the function body, we write:
function returnValue(argument) {
return argument;
}
Now, a function is something you make, so you decide what happens inside the function. You can give it any task you want, but in this case, we are just returning the argument we gave it.
Now, for task 2, it wants you to make a new variable called "echo". So, underneath the function we just created:
function returnValue(argument) {
return argument;
}
var echo =
Then, it wants you to assign the function you just created to the var we are creating and pass in a string as the argument. Now here is where an argument comes in handy. You can skip an argument in a function alltogether if you are making a simple function that would just return a string we could not alter while calling the function. That would look something like this:
function simpleString() {
return "This is a string";
}
As you can see, i left the parentheses empty, so we did not give it an argument but we cannot alter the argument neither. So, when i call the function on the echo var, like this:
var echo = simpleString();
The contents of the echo var will always be the string "This is a string" we just gave it.
But, we made a function with an argument, so we can give it something we want to be stored in the echo var! So, we have:
function returnValue(argument) {
return argument;
}
var echo = returnValue("We are returning a String!");
We used the argument we created earlier to give something to the function while we are calling it. So, the var echo now contains the string "We are returning a String!". But if we would have given it something else, the echo var would have contained that input.
An argument can be named anything you want basically and it will be replaced by anything you give it while calling the function.
Hope i made it a but clear for you!
Elian
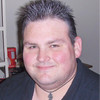
Roy Penrod
19,810 PointsHey, Hannah.
What confuses you about functions?
Here's what they're wanting for you from the challenge.
function returnValue(argument) {
return argument;
}
var echo = returnValue("My string.");
Starting with the "var echo" statement, here's what you're doing:
(1) Calling the function returnValue() and passing it a string containing the words "My string."
(2) The returnValue() function takes "My string." and stores it in a temporary variable called argument.
(3) The returnValue() function then immediately returns the contents of the variable argument. In this case, argument = "My string."
(4) The value returned from returnValue() function gets stored in the variable echo. In this case, echo = "My string."
Did that help?
Kristopher Van Sant
Courses Plus Student 18,830 PointsKristopher Van Sant
Courses Plus Student 18,830 PointsI've removed Krondor The Destroyers answer since it was not actually an answer and had nothing to do with what your working on. EDIT: Tried removing the answer, and they posted it again. My apologies Hannah.