Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial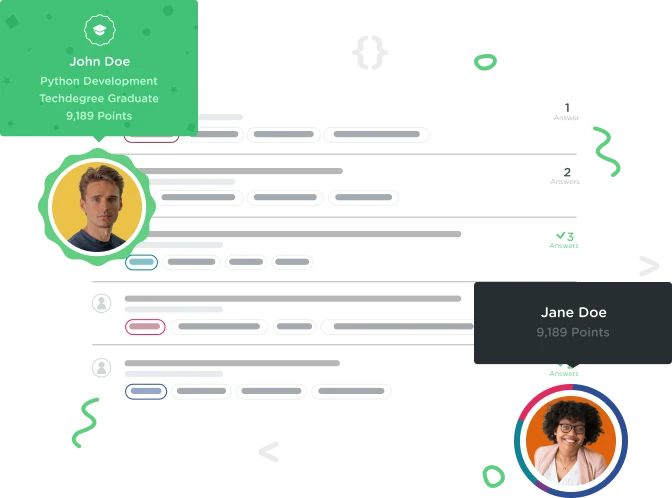
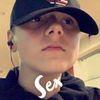
Keegan Swanson
1,339 PointsI am so so very much confused... Can someone please help me.
I've tried so many things for the top half but nothings working. I was told the bottom half was right, is it?
class Student:
name = "Your name"
def praise(self.name):
return
keegan = Student()
keegan.name = "Keegan"
print(keegan.praise())
3 Answers
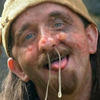
Josh Klink
2,330 PointsYo Keegan, you are way overthinking it.
class Student:
name = "Joshua Klink"
me = Student()
print(me.name)
As you can see, I used my name as an example you should use yours!!!
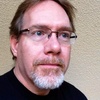
Chris Freeman
Treehouse Moderator 68,423 PointsYour structure isn't quite right.
- a method should have
self
as the first parameter. This becomes a reference to the instance executing the method. - using
self.name
is the correct way to reference the instance variable. Great job! - The
return
statement has no argument and will returnNone
. - The
return
statement argument should be a string that referencesself.name
Post back if you need more help. Good luck!!
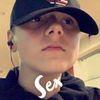
Keegan Swanson
1,339 PointsWhat do you mean when you say "the first parameter"?
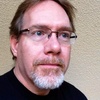
Chris Freeman
Treehouse Moderator 68,423 PointsWhen defining a method, the first item listed in the parameter list is the reference for the instance:
>>> class ClassName:
... def method_name(self, param_a, param_b):
... return f"result: param_a is {param_a}, param_b is {param_b}"
...
>>> class_instance = ClassName()
>>> method_result = class_instance.method_name("arg1", "arg2")
>>> method_result
'result: param_a is arg1, param_b is arg2'
The parameter self
is used during the instantiation of the instance and is not provided by the calling statement
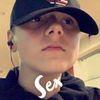
Keegan Swanson
1,339 PointsSo what would I do?

Howard Hufford
1,450 PointsHere are the directions for anyone reading:
I need you to add a method name praise. The method should return a positive message about the student which includes the name attribute. As an example, it could say "You're doing a great job, Jacinta!" or "I really like your hair today, Michael!".
In your example, Keegan, you started out correctly by adding the method praise. In this case we need to add (self) only afterwards.
class Student:
name = "Your Name"
def praise(self):
# (self) is the parameter used in methods and it will need to be referenced later in the code somewhere.
# So now that we have the method (def praise(self)) created, it needs to return a message and the name attribute.
return "Great job, {}!".format(self.name)
# Above, the parameter self was used to reference the name attribute. The printed message uses the format of self.name to access "Your Name"
What you have at the bottom is not necessary. If you wanted to add your name, you would replace "Your Name" with "Keegan"