Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial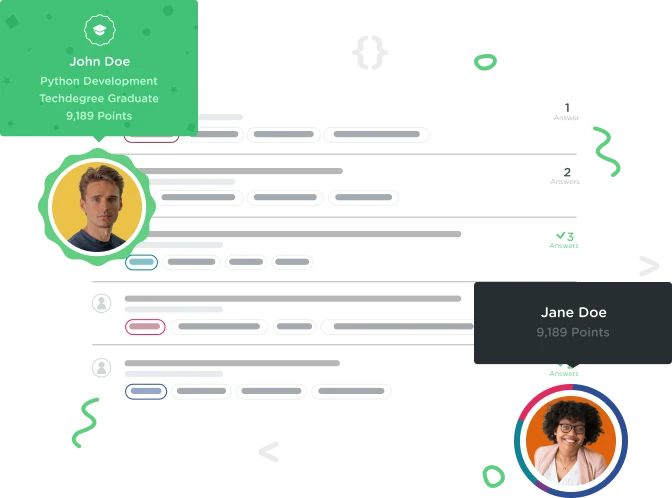
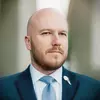
Jared Armes
Courses Plus Student 6,391 PointsI am so terribly stuck. I have no clue what to do here.
I have watched the video about 4 times now, and I still have no idea what to do. The challenge wants me to format the output in a way that is graphically pleasing; yet I have no idea how to go about doing that. Any help would be very much appreciated
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
add_transaction("Beginning Balance", 0)
end
def balance
balance = 0
@transactions.each do |transaction|
balance += transaction[:amount]
end
balance
end
def debit(description, amount)
add_transaction(description, -amount)
end
def credit(description, amount)
add_transaction(description, amount)
end
def add_transaction(description, amount)
@transactions.push(description: description, amount: amount)
end
end
1 Answer
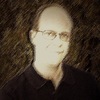
Jason Anders
Treehouse Moderator 145,860 PointsHi Jared,
I think you may just be over-thinking the challenge. The video does explain the simple answer, but is mixed in with other formatting and methods. This challenge, however, just wants you to create a to_s
method to print the name and balance.
After the end
for the add_transaction
method, but before the final end
of the code is where you will place your to_s
method.
def add_transaction(description, amount)
@transactions.push(description: description, amount: amount)
end
def to_s
"Name: #{name}, Balance: #{balance}" # These 3 lines are what is needed for this challenge
end
end
Hope that helps. Keep Coding! :)
Jared Armes
Courses Plus Student 6,391 PointsJared Armes
Courses Plus Student 6,391 PointsWow, thanks Jason! I was definitely over-thinking this issue, but then again, I figured that if I wasn't thinking about every little detail at once, I would miss something important. Ironically, it seems that I have!