Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial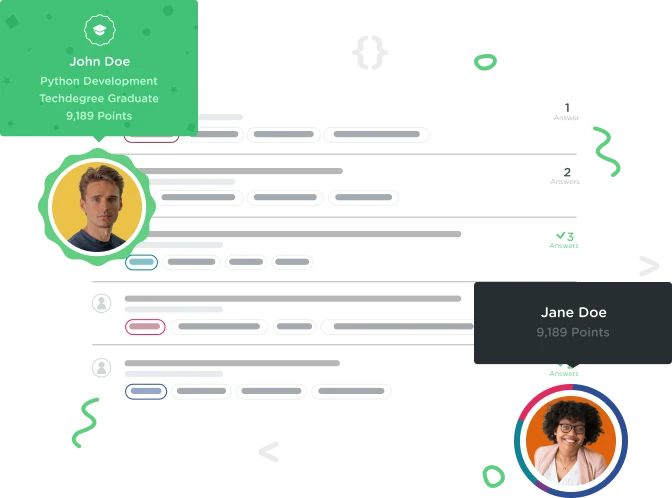
Andrew Nicholson
2,474 PointsI am struggling with the syntax of this code challenge
I'm struggling a lot with JavaScript, and finding I'm spending inordinate amounts of time on some of these simple code challenges - I literally have no idea where I should be adding the string in this challenge.
function returnValue( hello ) {
return hello;
var echo = returnValue(hello('hello'));
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Chris Shaw
26,676 PointsHi Andrew,
Your code is almost correct with the following exceptions:
- You have your
echo
variable declared within yourfunction
, it should be declared after it - You're attempting to call another undeclared function called
hello
- You should be passing My argument as the argument for
returnValue
The final code you should have is the following, again you were very close.
function returnValue(hello) {
return hello;
}
var echo = returnValue('My argument');
Happy coding!
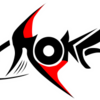
Justin Black
24,793 Pointsfunction returnValue( hello ) {
return hello;
var echo = returnValue(hello('hello'));
}
In your current implementation, you have created a function within it, that takes a parameter. Within that method you are instantly returning the value of the parameter. Then, after the function is done, you are trying to call the function, from within itself, to set the return value to a variable.
Spelled out in english like that, I'm sure you can now see where your error. If not, here's how it works.
When we create a function that takes an argument, that argument becomes a local variable for use within that function. When designing a function, we need to first decide what it should do. And then decide if that function should return any data.
Once we know the problem our function solves, and we know if it's returning data we can move forward. In this case, we know that our function is going to take an argument, and needs to pass the value of that argument back. So with this we know 2 things. 1. Our function takes an argument, probably in the form of a string. and 2. Our function does need to have a return.
Since we know these two things, and we know that any processing done to the data within it needs to happen before the return ( in our case none ) we can write our function like so:
function returnValue(hello) {
return hello;
}
So, there is our function done. Now how do we call this function? We know that this function returns data back. So it makes sense that we may want to store this data for future use within a variable like so:
var echo = returnValue("Hello World!");
Hope it helps
Andrew Nicholson
2,474 Pointsthanks guys, much appreciated