Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial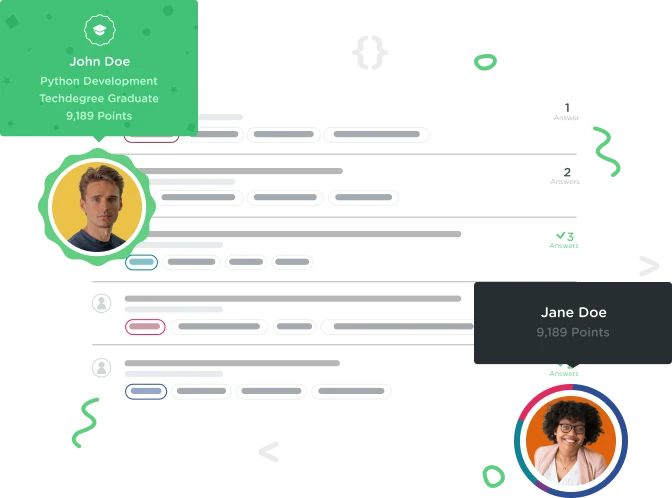

Steve Burgos
2,759 PointsI am struggling with understanding how to set up a method using strings rather than integers
This is my current code but I am having trouble understanding how to create the method using the string that I have and the structure for that string.
struct Person {
let firstName: String
let lastName: String
func getFullName ( first: String, last:String) -> [Person]{
var result: [Person] = first + last
}
}
1 Answer
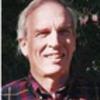
jcorum
71,830 PointsThe function doesn't need any parameters because it has access to the struct's member variables. It does need to show that it returns a String, so you need -> String after the function name. Inside the function you need to return a String. And in that String you use String interpolation to include the values of the two member variables.
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: "Steve", lastName: "Burgos") //use the Person struct to create a Person object
let fullName = aPerson.getFullName() //use the Person object to call the getFullName() method