Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial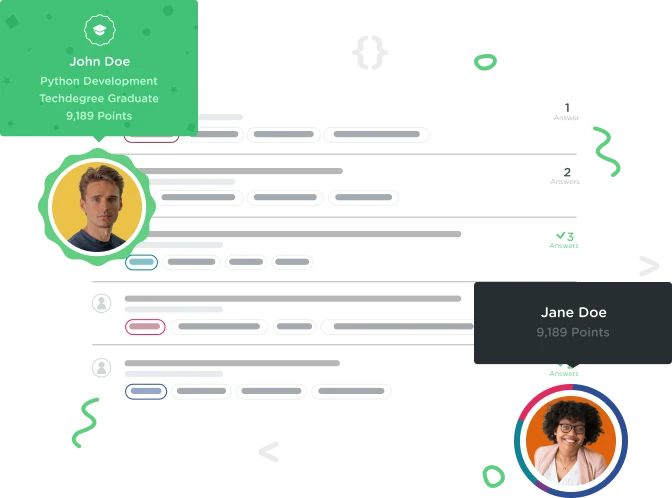

Chigozie Ofodike
11,197 PointsI am stuck and perplexed
I'm not too sure what i am supposed to do here with the second part of this challenge. If i didnt do the first part correctly, please let me know
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dictionary: [String : String]){
return nil
}
}
1 Answer

Joe Beltramo
Courses Plus Student 22,191 PointsYou need to validate that the dictionary has the minimum required items to be able to set the values in the struct, otherwise return nil.
The only two properties that are required in this case are title
and author
so those are the only two to guard against:
guard let title = dict["title"], let author = dict["author"] else {
// If title and author do not exist, the dictionary is missing required items, so return nil
return nil
}
// If title and author have been set, set all properties, if price and pubDate do not exist they will be set to nil which is acceptable as they are optional
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
Chigozie Ofodike
11,197 PointsChigozie Ofodike
11,197 Pointswhy the title/author? it isnt an optional type. I thought we only had to guard against optional types
Joe Beltramo
Courses Plus Student 22,191 PointsJoe Beltramo
Courses Plus Student 22,191 PointsIt depends on what you are trying to do. In this case, since
title
andauthor
are not optional types for the Struct, they are required, so you are guarding to ensure they exist, because if they do not exist, you do not want to create the Struct as setting a non-optional type to nil will result in a compile error.You want to guard against optional types when you dive into them so that you are not trying to access a property within a
nil
value.So here you are not guarding against a non-optional type, you are just making sure the non-optional types exist.
It is the same as saying:
IF dict["title"] does NOT exist OR dict["author"] does NOT exist then return nil ELSE let title = dict["title"] and let author = dict["author"]
Does that help clarify?