Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial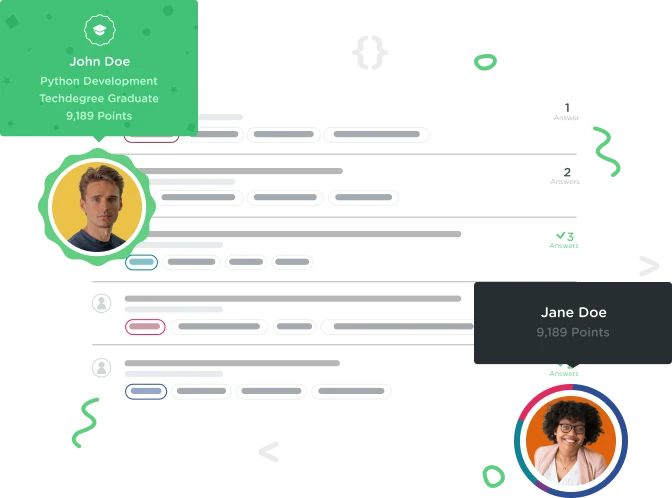

Randell Purington
9,992 PointsI am stuck and would like some assistance and a little explanation to why I am getting it wrong. please & Thank you
In this task, we have an array of numbers and we want to compute the sum of its values.
We have a variable ,sum, that will store the value of the sum of numbers from the array.
We also have a variable ,counter, which we will use to track the number of iterations of the while loop.
Step 1: Create a while loop. The while loop should continue as long as the value of counter is less than the number of items in the array. (Hint: You can get that number by using the count property)
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while (counter < numbers.count) {
sum += numbers[counter]
counter ++
}
1 Answer

Chris White
2,308 PointsHey Randell
You've almost got it right, there's just one minor syntax error on your counter incrementer. Swift 4, unlike a bunch of other extended c-like languages you may be used to no longer has a var++
increment (or var--
decrement) operator, instead you need to use counter += 1
the same way that you're summing the array numbers.
What might add to the confusion here is that earlier versions of Swift did include the ++
increment and --
decrement operators but they were deprecated and removed from Swift 3. You can read reasons why these were removed in the proposal by Chris Lattner, the creator of Swift on the Swift repo. The gist is that those operators add unnecessary complexity with limited gain (var += 1
isn't much longer than var++
) and were removed to simplify things.
Here's the code I used when completing that challenge:
while counter < numbers.count {
sum += numbers[counter]
counter += 1
}
You might also note that while adding parentheses around the condition is valid it is unnecessary and should probably be omitted. All of the control flow examples on Apple's Swift Language guide omit parentheses.
Typically, you'd only use parentheses in a conditional statement when you need complex boolean logic for multiple conditions like this:
var amAwesome = true
var noReally = true
while counter < numbers.count && !(amAwesome == false || noReally == false) {
sum += numbers[counter]
counter += 1
}
This example is silly but it demonstrates inverting the values of both variables in a single step. The conditionals chapter in We ❤ Swift has some great easy-to-digest information on conditionals if you want to dig in further.
Richard Barquero
474 PointsRichard Barquero
474 PointsHey Chris or anybody who would be kind to help. Break down for me the code in the while loop being recommended as the solution. I have tried entering this but to no avail. Why would counter be placed in square brackets as if making it an array?
while counter < numbers.count { sum += numbers[counter] counter += 1 }