Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial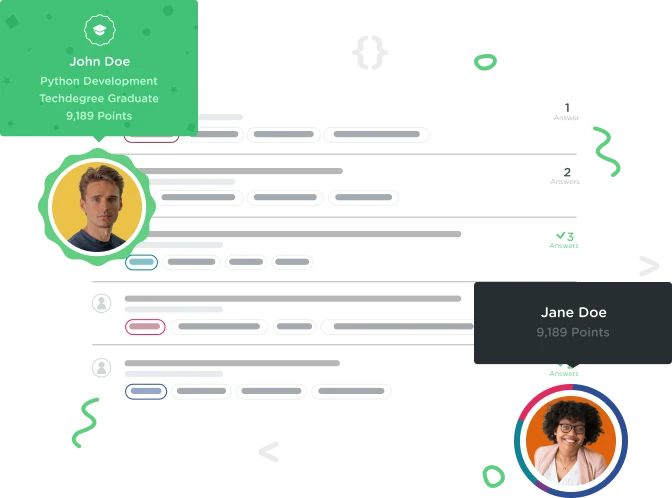

Merianna Kachadurian
904 PointsI am stuck, how could i write the following method in Java
in a task it asks to write the following method after i was done from the previous task: Great! Now let's create a similar method named isFullyCharged that checks to see if the current bar count is at the maximum charge. and bellow you can see the whole class including isFullyCharged method. what's the wrong? tried to write in another ways but they did not work!
GoKart.java
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
public GoKart(String color) {
this.color = color;
barCount = 0;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty(){
if (barCount == 0){
return true;
}
else return false;
}
public boolean isFullyCharged(){
if (charge()==0){
return true
}
}
}
```Java
5 Answers

Price Rebel
306 PointsThis is even simpler
public boolean isFullyCharged() { return MAX_BARS == barCount; }

Merianna Kachadurian
904 Pointsit worked for me as the following: public boolean isFullyCharged(){ if (barCount==MAX_BAR){ return true; } return false; }
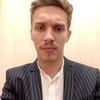
Adinel Berehorschi
1,771 PointsWorked for me as well, but you wrote "MAX_BAR" instead of "MAX_BARS".
public boolean isFullyCharged(){ if (barCount==MAX_BARS){ return true; } return false; }
That's the correct version. :)

terrimodomo
6,153 PointsNot sure what the problem is here for isFullyCharged????not working, please any ideas, thanks.
class GoKart { public static final int MAX_BARS = 8; private String color; private int barCount;
public GoKart(String color) { this.color = color; }
public String getColor() { return color; }
public void charge() { barCount = MAX_BARS; }
public boolean isBatteryEmpty (){ if(barCount == 0){ return true; } else{ return false; } } } public boolean isFullyCharged(){ if (barCount == MAX_BARS){ return true; } return false; }

Ajit Shrestha
3,149 Pointspublic boolean isFullyCharged(){ if (barCount == MAX_BARS){ return charged; } else { return notCharged; } } this worked for me

Jonathan Hector
5,225 PointsIt's actually a simple error Remember that your barCount is being updated through the charge() method. So what you need to do is just call it and see where it is up to. Simple way to view a Boolean is to just think of a way to check if something is 'true' or 'false'. Just instantiate a variable that does the job and don't forget the ';'. Also you are checking the barCount not the actual 'charge()' method. Hope that helps
public boolean isFullyCharged(){
boolean charged = false;
if (barsCount == 0){
return true;
}
return charged;
}

Ivan Bagaric
Courses Plus Student 12,356 PointsYeah, but also he can do shortly:
public boolean isFullyCharged(){ return (barsCount == 0) ? true : false; }