Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial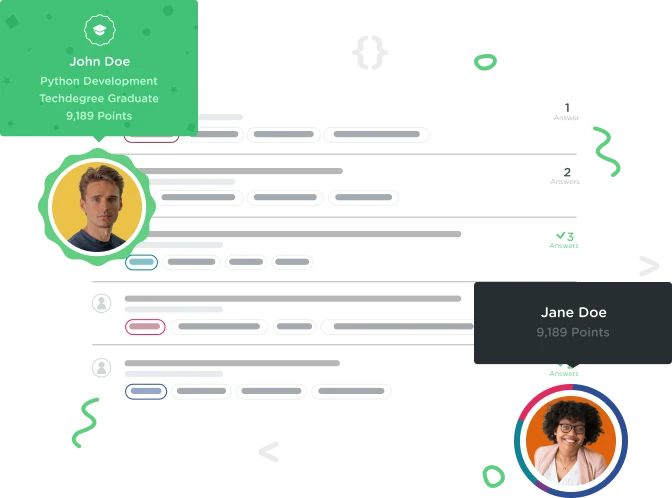

Tomas Pavlik
26,726 PointsI am stuck - if else statement at the end
Hi people, I am trying to add the part of the code that writes a message "Student not found" if the user writes student's name which is not on the list. I've added an else if statement at the end, but something is wrong. The program keeps sending "Student not found", no matter what i write. Thanks for advice. P.S. Hope my english is understandable :-)
My code:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Type the students name or quit to exit the program.");
if (search === null || search.toLowerCase() === "quit") {
break; }
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search) {
message = getReport( student );
print(message);
} else if (student.name !== search) {
print("Student not found!");
}
}
}
4 Answers
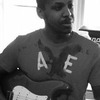
Samuel Webb
25,370 PointsAll I did was change it to an else statement and it worked. Like this:
if (student.name === search) {
message = getReport( student );
print(message);
break;
} else {
print("Student not found!");
}
I also added a break statement after the name. But that was just because I wanted to stop the program after I found a name. Adding a break statement right before the end of your while loop will also prevent you from falling into the trap of an infinite loop while you're testing your program. You can remove it when you think things are working properly.

Tomas Pavlik
26,726 PointsHi Samuel, thank you, it seems adding the break statement solved the problem. I kept my previous version of if else with this comparison: (student.name !== search) and just added the break statement to the if and ot works... But I'm still confused why the programm did not work properly without the break statement. In my view, if the if condition is true and the code is executed, the else if or else statement should be skipped...
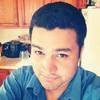
Jorge Felico
15,543 PointsHi Tomas, your student variable is empty...Shouldn't it be an array with a list of student names?
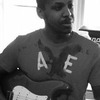
Samuel Webb
25,370 PointsThere's a students array coming from another file. He set the student variable blank at the beginning because it is what houses each student down in the for loop.

Tomas Pavlik
26,726 PointsHi Jorge, sorry, I forgot the first JS file which contains the list of student names. everything forks fine, until i add the last else if statement...

Tomas Pavlik
26,726 PointsThank you for help Samuel :-) This was the first time I've written on the forum, so I am not too familiar with all the funcionalities... :-)

Isaac Minogue
3,543 PointsI spent so long on this... But thanks to Tomas Pavlik & Samuel Webb 's comments, I saw the break statement used so I gave it a try. I already had it in the 'else if' clause, but not in the 'if' clause. As conditional statements are basically "either this happens, or this happens" (never both) – is that why both need a break?
ie. in my scenario, when the response was a valid name, it would jump to the end of the statement and just keep repeating printing "No student named ___ was found. Try another search," every time – no matter if it was correct or not.
All you pros out there – Is that right?
var message = '';
var student;
var response;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
response = prompt("Search student records: type a name or type 'quit' to end");
if (response.toUpperCase() === 'QUIT' || response === null) {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name.toUpperCase() === response.toUpperCase() ) {
message = getStudentReport(student);
print(message);
break;
} else {
message = "<p> No student named '" + response + "' was found. Try another search</p>";
print(message);
}
}
}
now onto the next challenge...

Josh Aharonoff
2,613 PointsHey - does anyone have an input on why there is a break needed for this answer? I actually ran into the same problem. The way I saw it - the code should ask the user for a name continuously until "quit" is typed in or cancel is pressed.
When the code runs, it should first check if the search value is equal to the student.name object. If it is, no need to jump to the elseif statement(since the condition was satisfied), and start the question again.
If the search is not equal to the student.name object, then return a value staying it was not found, and start the question again.
While the "break" solves this problem, I'm really confused why. Shouldn't the if statement by default break when the first condition is satisfied?

max zimmermann
19,309 PointsI think the break is necessary because it is a for loop. The only condition that will stop the loop is if i < students.length. When I had this problem the only name that I could get to print was 'Trish' because in the array of objects I had she was the last one, so when the loop ended that if condition (search === student.name) was the only one that would evaluate as true. That's my best guess though.
Samuel Webb
25,370 PointsSamuel Webb
25,370 PointsHello Tomas,
I edited your question, adding better formatting to make your code more readable. In the future, make sure you use the syntax in the Markdown Cheatsheet which is at the bottom of the Add Comment and Add an Answer boxes.