Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial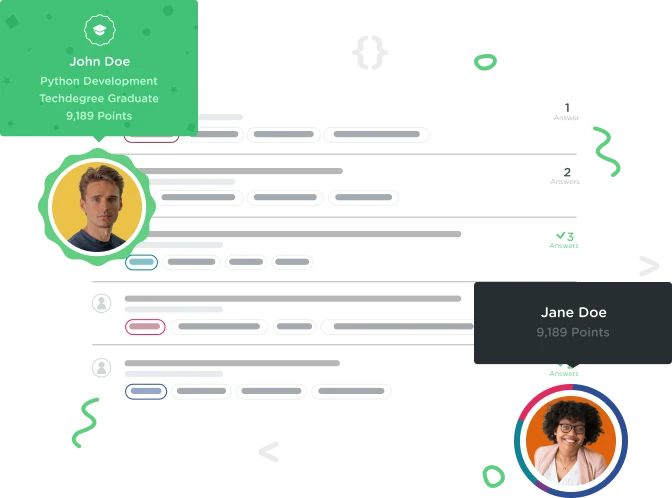

David Sciuto
753 PointsI am stuck in an infinite loop and can't seem to figure out why.
I am working on trying to follow along with the video and something seems to have broke along the way. I have went through countless times and cant find the problem and was hoping someone could help.
Here is the code I am using.
public class Hangman {
public static void main(String[] args) {
// Your incredible code goes here...
Game game = new Game ("treehouse");
Prompter prompter = new Prompter(game);
while (game.getRemainingTries() > 0) {
prompter.displayProgress();
prompter.displayProgress();
}
}
}
class Game {
public static final int MAX_MISSES = 7;
private String answer;
private String hits;
private String misses;
public Game(String answer) {
this.answer = answer;
hits = "";
misses = "";
}
public boolean applyGuess(char letter) {
boolean isHit = answer.indexOf(letter) != -1;
if (isHit) {
hits += letter;
} else {
misses += letter;
}
return isHit;
}
public int getRemainingTries() {
return MAX_MISSES - misses.length();
}
public String getCurrentProgress() {
String progress = "";
for (char letter : answer.toCharArray ()) {
char display = '-';
if (hits.indexOf(letter) != -1) {
display = letter;
}
progress += display;
}
return progress;
}
}
import java.util.Scanner;
class Prompter {
private Game game;
public Prompter(Game game) {
this.game = game;
}
public boolean promptForGuess(){
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a letter: ");
String guessInput = scanner.nextLine();
char guess = guessInput.charAt(0);
return game.applyGuess(guess);
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s%n",
game.getRemainingTries(),
game.getCurrentProgress());
}
}
1 Answer
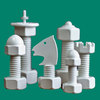
Steven Parker
231,268 PointsThe main loop in Hangman.java only displays the progress (twice each time!):
while (game.getRemainingTries() > 0) {
prompter.displayProgress();
prompter.displayProgress();
}
Since it doesn't do anything that would change the remaining tries, it continues forever.
But in the video code, the main loop displays the progress, and then gets another guess:
while (game.getRemainingTries() > 0) {
prompter.displayProgress();
prompter.promptForGuess(); // <-- get a guess (and change remaining tries)
}

David Sciuto
753 PointsThanks Steven, honestly sometimes it helps having a second pair of eyes looking at something. I swear I was looking at this for 20 minutes and didn't see anything wrong haha.
David Sciuto
753 PointsDavid Sciuto
753 PointsThe only thing the loop is doing is displaying progress, but in the video Craig seems to have the same code but with a different outcome.