Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial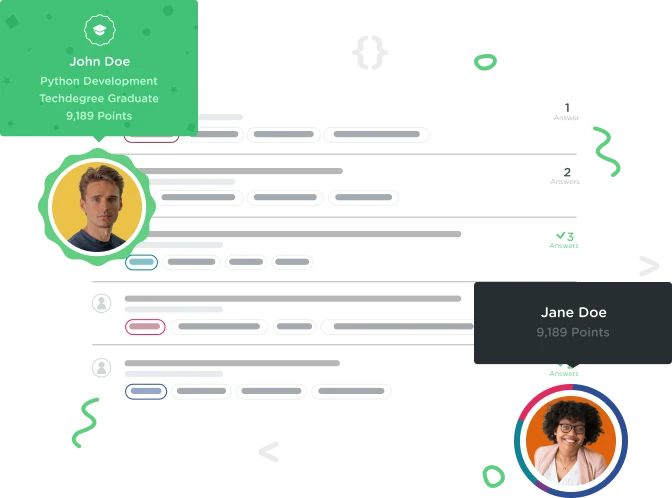
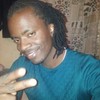
Adlight Sibanda
5,701 Pointsi am stuck on remaining tries
Add a new method that returns the remaining characters available, based on the length of what is currently stored in mText. were have i gone wrong seriously.... thank u in advance.
public class Tweet {
public final static int MAX_CHARACTER_LIMIT = 140;
private String mText;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
public int getRemainingCharacters() {
return MAX_CHARACTERS - mText.length();
}
}
10 Answers
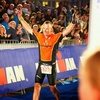
Steve Hunter
57,712 PointsYou're correct - but you didn't use your constant correctly.
public int getRemainingCharacters() {
return MAX_CHARACTER_LIMIT - mText.length();
}
That should do it for you.
Steve.
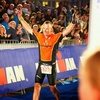
Steve Hunter
57,712 PointsHi Hannah,
There seems to be something wrong with the challenge you're on so let's go right back to the beginning and walk through this. Refresh the browser page to restart the challenge. You start with this code:
public class Tweet {
private String mText;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
}
The question is: Please add a new constant to store the maximum number of characters allowed which is 140. Use the proper access level modifiers to make it unchangeable and accessible right off of the class. Follow the proper naming convention.
So, for that you use the ALL_CAPS naming convention and add a line right at the top of the class:
public class Tweet {
public final static int MAX_CHARACTER_LIMIT = 140;
.
.
The rest of the code is the same. Next, the question states: Add a new method that returns the remaining characters available, based on the length of what is currently stored in mText.
That is where you've met with some challenges. We're creating a new method, so that's inside the class' curly braces and outside of any of the other braces in there:
public class Tweet {
public final static int MAX_CHARACTER_LIMIT = 140;
private String mText;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
// let's put it here!
} // <-- must be before this
The method is public
it returns an int
and takes no parameters, and is called getRemainingCharacters
. That looks like:
public int getRemainingCharacters() {
// do some stuff in here
}
Inside the method we subtract from the CONSTANT we created first, the length of the mText
member variable. We get that by chaining the .length()
method to the variable. We want to return
the result of that:
public int getRemainingCharacters() {
return MAX_CHARACTER_LIMIT - mText.length();
}
That goes inside the class where we said above, so your final code looks like:
public class Tweet {
public final static int MAX_CHARACTER_LIMIT = 140;
private String mText;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
// let's put it here!
public int getRemainingCharacters() {
return MAX_CHARACTER_LIMIT - mText.length();
}
}
That should work for you - I tested it here! Let me know how you get on. And sorry for the long answer - I wanted to make sure you had everything right.
Steve.
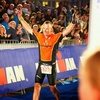
Steve Hunter
57,712 PointsThe error you mention is caused when the method is put outside the class - I just replicated that here. So, move your method inside the last curly brace of the class and it should work fine - or it'll throw a different error.
Keep me posted.
Steve.
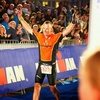
Steve Hunter
57,712 Points./Tweet.java:16: error: class, interface, or enum expected
public int getRemainingCharacters() {
^
./Tweet.java:18: error: class, interface, or enum expected
}
^
2 errors
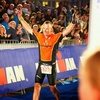
Steve Hunter
57,712 PointsOK - so you've declared the constant called MAX_NUM_CHARS
at the top of the class, that's fine. Then, when you've come to use it, you've used the MAX_CHARACTER_LIMIT
name that I used in my example. You'll need to use an identical name in both places as they are referring to the same thing.
So, either:
public class Tweet {
private String mText;
public static final int MAX_NUM_CHARS = 140; // this variable
.
.
.
public int getRemainingCharacters() {
return MAX_NUM_CHARS - mText.length(); // used here; same name
}
}
or this:
public class Tweet {
public final static int MAX_CHARACTER_LIMIT = 140; // same name here
private String mText;
.
.
.
// let's put it here!
public int getRemainingCharacters() {
return MAX_CHARACTER_LIMIT - mText.length(); // and here
}
}
So, be consistent and use the same variable name in both places - decide which one you want to use; it doesn't matter - and you're done!

Hannah Flynn
21,429 PointsNot really. That code states 2 errors: on the i of the "int" & on the "}". I only know that you need to delete int and these breackets: { }. The only mistake is on the "getRemainingCharacters" Can you find the correct way to fix the mistake?
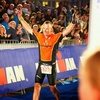
Steve Hunter
57,712 PointsHi Hannah,
Can you post your code please?
Thanks,
Steve.

Hannah Flynn
21,429 PointsSteve, this is the code: public getRemainingCharacters() return MAX_CHARACTERS - mText.length();
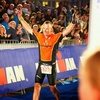
Steve Hunter
57,712 PointsHi Hannah,
You need to open a pair of curly braces after the first line and then close the pair after the return line. Like this:
public int getRemainingCharacters() { // <- open the {
return MAX_CHARACTER_LIMIT - mText.length();
} // <- close the }
I hope that helps - let me know how you get on.
Steve.

Hannah Flynn
21,429 PointsThat didn't help. The codes states 2 syntax errors: on the int and the curly 2nd curly brace. Can you find the correction on the 2 errors for the code.

Hannah Flynn
21,429 PointsYou did good except for 1 error the code found out on now: MAX_CHARACTER_LIMIT. Can you find out the correct way? The code I did looks like this: public int getRemainingCharacters() {
return MAX_CHARACTER_LIMIT - mText.length();
}
}
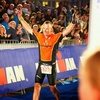
Steve Hunter
57,712 PointsCan you post your whole code - just copy & paste it in.
The MAX_CHARACTER_LIMIT
should be fine if it is named that way both times; first when it is declared and second when it is used.

Hannah Flynn
21,429 PointsYou got it. The code I did looks like this:
public class Tweet {
private String mText;
public static final int MAX_NUM_CHARS = 140;
public Tweet(String text) {
mText = text;
}
public String getText() {
return mText;
}
public int getRemainingCharacters() {
return MAX_CHARACTER_LIMIT - mText.length();
}
}
And one more thing, I will post you the preview to help you see what i mean:
./Tweet.java:14: error: cannot find symbol
return MAX_CHARACTER_LIMIT - mText.length();
^
symbol: variable MAX_CHARACTER_LIMIT
location: class Tweet
1 error
The ^ is pointing on the M.

Hannah Flynn
21,429 PointsThat helps. Thank you.
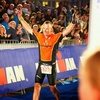
Steve Hunter
57,712 PointsNo problem - glad you got it fixed.
Adlight Sibanda
5,701 PointsAdlight Sibanda
5,701 PointsThank you Steve, it worked, have a good one.