Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial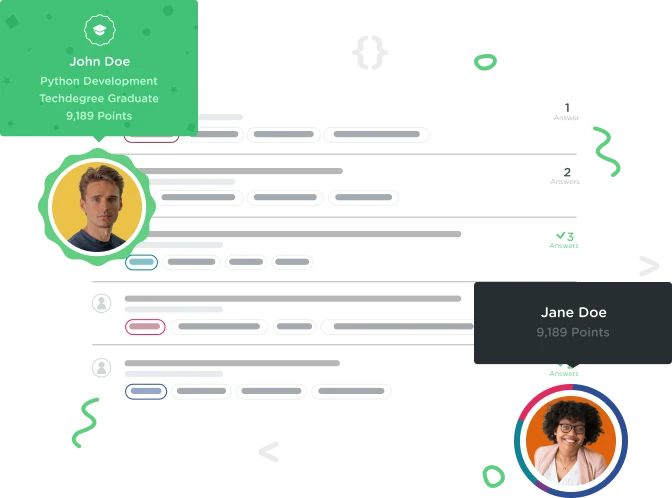

rodnel lighty
1,090 PointsI am stuck on the ScarbblePlayer class. I created the getCountOfLetter method and can not get it to return the right #.
How do i get it to increment with each letter?
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) {
int tileCount = 0;
for (char item : tiles.toCharArray()) {
int count = 1;
if (tiles.indexOf(item) == letter) {
count++;
}
tileCount += count;
}// End of for
return tileCount;
}// End of method
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer

Sondre Dahl
10,086 PointsThe error lies in your for loop. After the first execution of the loop, the value of tileCount will be 1 because you are adding your count to tileCount in every iteration. Right now you are incrementing count and adding the count value to tileCount. However, this means that when count = 3 you will be adding 3 to your tileCount even though you have only found 2 letters. Look at the comments in the code below:
public int getCountOfLetter(char letter) {
int tileCount = 0; // This is the value that you wish to increment inside the loop
for (char item : tiles.toCharArray()) {
int count = 1; // This variable isn't really necessary because you can use
//tileCount in your loop as long as you declare it
//outside of the loop.
if (tiles.indexOf(item) == letter) {
count++; // This should be changed into tileCount as
//tileCount is the variable you wish to increment.
}
tileCount += count; // Before you have found any matching
//letters, this will be set to one.
//It will add one for every iteration because count
//is set to 1 and you add count to tileCount every
//loop.
}// End of for
return tileCount;
}// End of method
I hope this clarified things :) This is my first time answering a question so if anything is unclear, tell me and I will try to make it clearer!
rodnel lighty
1,090 Pointsrodnel lighty
1,090 PointsYou have explained it well enough to clear up my confusion. Thank you very much!!
Sondre Dahl
10,086 PointsSondre Dahl
10,086 PointsYou're welcome! :)