Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial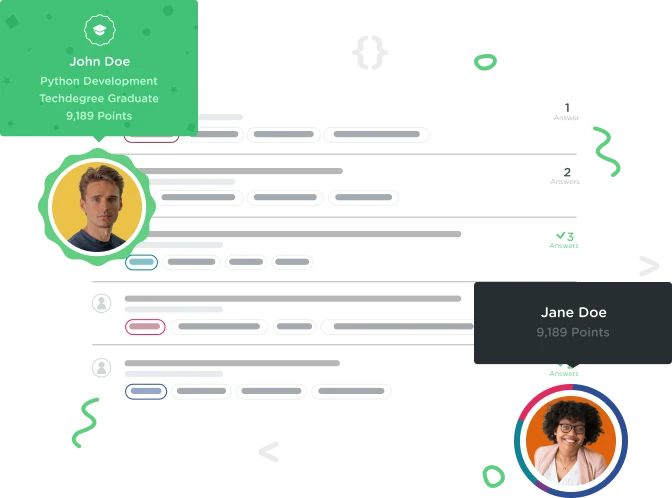
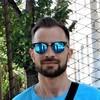
Oszkár Fehér
Treehouse Project ReviewerI am stucked here a little bit, can someone help me out a little please?
i am not fully understand the question and i am not sure what i should do next
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides = 20)
from dice import D20
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
def roll(self, size):
super().__init__(size=size, die_class=D20, *args, **kwargs)
@property
def total(self):
return sum(self)
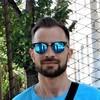
Oszkár Fehér
Treehouse Project ReviewerI am sorry for this mess, i tried to show you all the code but i have to left hands... Thank you that you didn't wrote me the code and you made me to do by myself, i learned more like this because i don't like to give up.
2 Answers
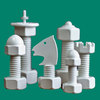
Steven Parker
231,269 PointsHere's a couple of hints:
- you don't need to override
__init__
, just implementroll
- since you'll be returning a new instance, this might be a good place for a classmethod
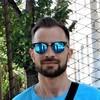
Oszkár Fehér
Treehouse Project Reviewerthis is the quiz: "Now update Hand in hands.py. I'm going to use code similar to Hand.roll(2) and I want to get back an instance of Hand with two D20s rolled in it. I should then be able to call .total on the instance to get the total of the two dice. I'll leave the implementation of all of that up to you. I don't care how you do it, I only care that it works"
i made with classmethod but this gives me back a list and it doesn't give me back the total and the total method it's already in the quiz. the quiz telling me that he can't find the length of Hand.
i am still stucked...
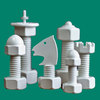
Steven Parker
231,269 PointsIf you return any kind of list it should be able to get the length. But you should return a Hand, which is a specific kind of list with extra methods (such as total).
Have you looked at some of the other questions in the forum asked about this challenge? This is a common subject of questions.
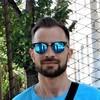
Oszkár Fehér
Treehouse Project Revieweri didn't find similar problem on the forum, it looks like only me i can't pass this test. i can get till Hand.roll(2) and even Hand.roll(2).total but probably something i don't do right because it's telling me that can't find the lenght of Hand.
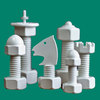
Steven Parker
231,269 PointsHave you made some changes so that roll now returns a Hand? If you have and are still getting that error, show that code as it is now (be sure to use markdown for code formatting).
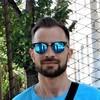
Oszkár Fehér
Treehouse Project ReviewerI made it finally, but i am still confused because after u wrote me that i should make classmethod , i wrote exacly the same way how it was now when i passed the test and it didn't let me pass, so i started to change the init function and the only change i made that i took out the "self.sort()". Anyway, thank you for your help, You was the only one who acctually answered me back.
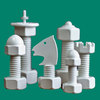
Steven Parker
231,269 PointsI'm not sure what you did, I don't see any "self.sort()
" in your code. Also, I would think you would have to return something in your roll
method for it to work. Would you mind showing me your final solution?
You don't have to use classmethod, but that's what I did.
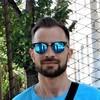
Oszkár Fehér
Treehouse Project Reviewerfrom dice import D20
class Hand(list):
def __init__(self, size=0, die_class=D20, *args, **kwargs):
super().__init__()
for _ in range(size):
self.append(die_class())
# self.sort()
@classmethod
def roll(cls, size):
return cls(size)
@property
def total(self):
return sum(self)
i am not sure this is the how i should show but i hope you can read. # self.sort()
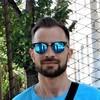
Oszkár Fehér
Treehouse Project Reviewerfrom dice import D20
class Hand(list):
def __init__(self, size=0, die_class=D20, *args, **kwargs):
super().__init__()
for _ in range(size):
self.append(die_class())
@classmethod
def roll(cls, size):
return cls(size)
@property
def total(self):
return sum(self)
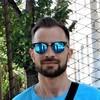
Oszkár Fehér
Treehouse Project Reviewerfrom dice import D20
class Hand(list):
def __init__(self, size=0, die_class=D20, *args, **kwargs):
super().__init__()
for _ in range(size):
self.append(die_class())
@classmethod
def roll(cls, size):
return cls(size)
@property
def total(self):
return sum(self)
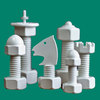
Steven Parker
231,269 PointsThose 3 marks for code quoting should be accents (```), not apostrophes ('''). But I get it, thanks.
I still don't know where that "self.sort()
" came from, but I can see by adding that return to your roll method that it would work. But it's a lot more complicated than the solution I used of just writing roll by itself.
Anyway, congratulations on solving it.

Stephen Cole
Courses Plus Student 15,809 PointsI had the same problems.
The self.sort() worked in the videos because we added all the magic methods needed to "do the math" required for sort to work.
Without them, the function didn't know what to do.
That was much harder than it needed to be.
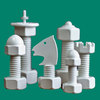
Steven Parker
231,269 PointsIt does say, "I don't care how you do it, I only care that it works.", which invites all sorts of colorful solutions.
But since you can implement roll
with just a few lines it seemed a much simpler approach than overriding __init__
.
Oszkár Fehér
Treehouse Project ReviewerOszkár Fehér
Treehouse Project ReviewerThank you.