Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial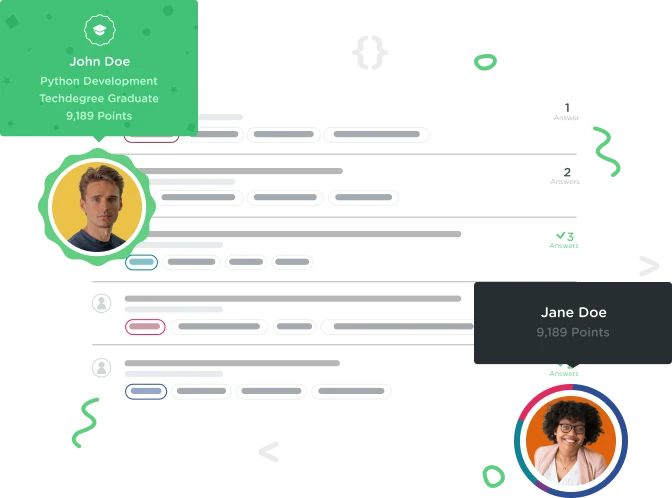

Joseph Bennett
529 PointsI am totally confused on the challenge. it displays something about not decrementing but I have.
Double check you are calling the original drive method and passing in 1.
public void drive() {
drive (mBarsCount--);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
Very confused
3 Answers

qlpxjevhuv
10,503 PointsThe challenge is expecting you to use the new technique you learned: method signatures. You already had a drive method that subtracts mBarsCount. Now you need to construct a new method that takes no parameters and passes in the value of 1 to the old drive method. By doing so, we avoid violating the D-R-Y principle.
public void drive() {
drive(X);
}
public void drive(int laps) {
mBarsCount -= laps;
}

Joseph Bennett
529 PointsI swear on every part I get stuck on I over complicate it. Thank you.
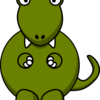
luis martinez
2,480 PointsThank you

Alvaro Gutierrez
1,471 PointsI am having problems with this same task. I also am getting "Something seems to be wrong with your method. Double check you are calling the original drive method and passing in 1." Here is my code:
public void drive() {
drive(mBarsCount);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
```
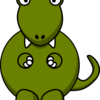
luis martinez
2,480 Pointswhat i did for the drive method was put
public void drive() { drive(1); }
check if it works

Alvaro Gutierrez
1,471 PointsThanks Luis! That worked
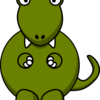
luis martinez
2,480 Pointsif you have problems with the next challenge look at this link https://teamtreehouse.com/forum/illegalargumentsexception

Alvaro Gutierrez
1,471 PointsOk, I appreciate it.
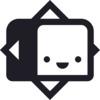
Jake Fleming
Treehouse Guest TeacherFor those of you still confused, here's what your code should look like:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}

Terence Evans
1,870 PointsThank you Jake. I'm still waiting for the lightbulb over my head to click on. One day, one day.
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi Joseph,
I add markdown to help make the code more readable. If you're curious about how to add markdown like this on your own, checkout this thread on posting code to the forum . Also, there is a link at the bottom called Markdown Cheatsheet that gives a brief overview of how to add markdown to your posts.
Cheers!