Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial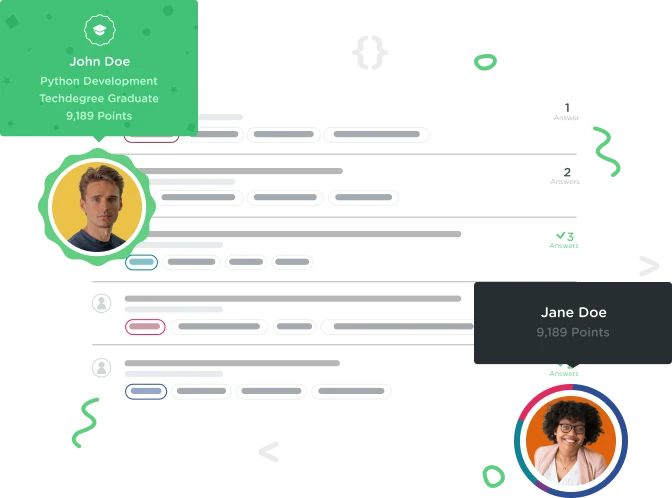

Humoyunmirzo Nosirov
Courses Plus Student 5,154 PointsI am totally stuck again , can anyone give me some help,
Using the filter and map methods on the todos array, create an array of unfinished task strings. See the comments below to see the correct result. Store the new array in the variable unfinishedTasks.
const todos = [
{
todo: 'Buy apples',
done: false
},
{
todo: 'Wash car',
done: true
},
{
todo: 'Write web app',
done: false
},
{
todo: 'Read MDN page on JavaScript arrays',
done: true
},
{
todo: 'Call mom',
done: false
}
];
let unfinishedTasks;
unfinishedTasks = todos
.filter(todos => {todos.done} !== 'false')
.map(todos => '${todos.todo}');
// unfinishedTasks should be: ["Buy apples", "Write web app", "Call mom"]
// Write your code below
4 Answers

Humoyunmirzo Nosirov
Courses Plus Student 5,154 Pointsconst todos = [ { todo: 'Buy apples', done: false }, { todo: 'Wash car', done: true }, { todo: 'Write web app', done: false }, { todo: 'Read MDN page on JavaScript arrays', done: true }, { todo: 'Call mom', done: false } ]; let unfinishedTasks; unfinishedTasks = todos.filter(todos => todos.done !== false) .map(todos => todos.todo);
did I miss smth

Juan Naputi
1,772 PointsYou're very, very close. Replace
todos.done !== false
with
todos.done === false
By having it as todos.done !== false
, you are grabbing the tasks from the array that you have completed. To grab the unfinished tasks, you would use the second one since it checks for all tasks that are not done.

Juan Naputi
1,772 PointsYou are on the right track. And, you are much closer than you think. Just some minor syntax and type issues.
For your filter() function, you do not need to have the brackets -- {} -- around your todos variable. When accessing an objects property, the dot (.) operator alone is enough. Remove the single quotes '' from your false value. Boolean types don't have to be surrounded by that. true and false is a value in-itself. By surrounding it with single-quotes, you turn the check from "is todos.done equal to false?" to "does todos.done equal the word 'false'?"
For your map, you can remove the single-quotes and the ${} from your todos.todo. Just like in the filter, you can access the properties of an object just using the dot (.) operator.
So, your fix would be:
unfinishedTasks = todos.filter(todos => todos.done !== false)
todos.map(todos => todos.todo)
Regarding objects, if there was the following object:
const me = {
name: 'Juan',
age: 24,
graduationYear: 2017
}
If you wanted to access any of the following properties (name, age, graduationYear), you simply just use the dot (.) operator.
me.name => 'Juan'
me.age => 24
me.graduationYear => 2017
And some extras, in case you are interested.
If you are dealing with a group of items (which is the array of todos), when calling filter() or map(), it is common to name the variable name as a singular version of the array.
So, for the array of todos, you could switch the name of your variable in the filter() function from:
todos.filter(todo => todo.done !== false)
Notice how I named the variable todo since this represents only one todo in the list
And for the map:
.map(todo => todo.todo)
This one is kind funny one since you have two todo names. But, you are basically saying: for this todo, give me the value of the todo property.
If it's a little confusing, don't worry about it. As I said, it is just some extras and I'm sure it will come over time if it hasn't already.
Anyhow, Just know that you were quite close on your answer. It will get easier as time goes on. Just keep on practicing it and it literally will be a breeze down the line.
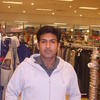
Muhammad Anwar Ul Haq
Full Stack JavaScript Techdegree Graduate 18,781 Pointsconst todos = [
{
todo: 'Buy apples',
done: false
},
{
todo: 'Wash car',
done: true
},
{
todo: 'Write web app',
done: false
},
{
todo: 'Read MDN page on JavaScript arrays',
done: true
},
{
todo: 'Call mom',
done: false
}
];
let unfinishedTasks;
// unfinishedTasks should be: ["Buy apples", "Write web app", "Call mom"]
// Write your code below
unfinishedTasks = todos.filter (todoos => todoos.done === false) .map( todoos => todoos.todo);```
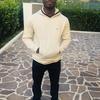
Mark Wisdom Reeves
Full Stack JavaScript Techdegree Graduate 18,275 PointsYou guys are very right. This also worked for me. Thanks for the ideas. Happy coding
todo.done !== true || false