Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial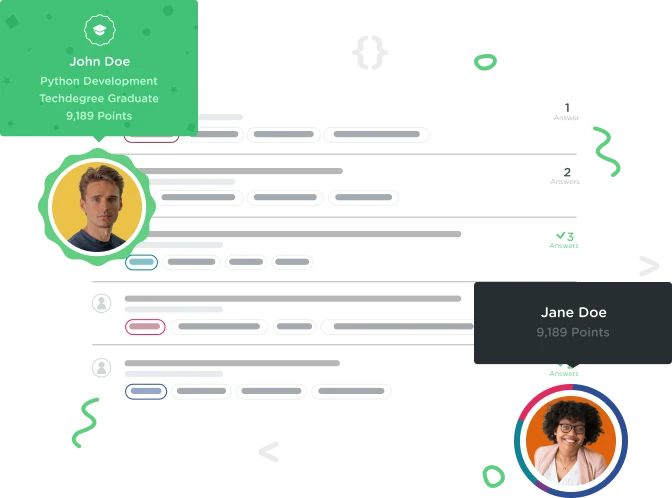

Adrian Cunanan
Front End Web Development Techdegree Student 9,996 PointsI am trying to build a simple calculator with Ruby. Using Ruby Basic topics. Please help...
Here is my code:
# This method greets the user on start up and request their name
# It returns their name
def greeting
name = gets "Hello! Please type your name: "
puts "It is nice to meet you #{name}. I am a simple calculator application. \n
I can add, subtract, multiply, and divide.\n"
return name
end
# This method ask the user what type of calculation they would like to perform
# It returns the operation or an error for erroneous entry
def request_calculation_type
operation_selection = gets "Type 1 to add, 2 to subtract, 3 to multiply, or 4 to divide two numbers: "
if operation_selection == 1
return "add"
elsif operation_selection == 2
return "subtract"
elsif operation_selection == 3
return "multiply"
elsif operation_selection == 4
return "divide"
else return "error"
end
# This method performs the requested calculation
# It returns the result of the calculation
def calculate_answer(operator, a, b)
if operator == "add"
return result= a + b
elsif operator == "subtract"
return result = a - b
elsif operator == "multiply"
return result = a * b
elsif operator == "divide"
return result = a / b
end
name = greeting
run_calculator = 1
while run_calculator == 1
current_calculation = request_calculation_type()
if current_calculation == "error"
puts "I do not understand this type of calculation... Can we try again?"
else
first_number = gets "What is the first number you would you like to #{calc_type}: "
second_numer = gets "What is the second number you would like to #{calc_type}: "
answer = calculate_answer(current_calculation, first_number, second_number)
puts "The answer is #{answer}"
run_calculator = gets "Type 1 to run another calcution or 2 to end: "
end
end
Here is the error:
calculator.rb:69: syntax error, unexpected end-of-input, expecting keyword_end
FYI - Line 69 is the end in the code
Please help... I would like to accomplish this as my first Ruby program ;-)
10 Answers
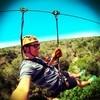
thomascawthorn
22,986 PointsI had a play and had more success with this:
# This method greets the user on start up and request their name
# It returns their name
def greeting
puts "Hello! Please type your name: "
name = gets
puts "It is nice to meet you #{name}. I am a simple calculator application. \n
I can add, subtract, multiply, and divide.\n"
name
end
# This method ask the user what type of calculation they would like to perform
# It returns the operation or an error for erroneous entry
def request_calculation_type
puts "Type 1 to add, 2 to subtract, 3 to multiply, or 4 to divide two numbers: "
operation_selection = gets.to_i
if operation_selection == 1
"add"
elsif operation_selection == 2
"subtract"
elsif operation_selection == 3
"multiply"
elsif operation_selection == 4
"divide"
else
"error"
end
end
# This method performs the requested calculation
# It returns the result of the calculation
def calculate_answer(operator, a, b)
if operator == "add"
a + b
elsif operator == "subtract"
a - b
elsif operator == "multiply"
a * b
elsif operator == "divide"
a / b
end
end
name = greeting
run_calculator = 1
while run_calculator == 1
current_calculation = request_calculation_type()
if current_calculation == "error"
puts "I do not understand this type of calculation... Can we try again?"
else
puts "What is the first number you would you like to #{current_calculation}: "
first_number = gets.to_i
puts "What is the second number you would like to #{current_calculation}: "
second_number = gets.to_i
answer = calculate_answer(current_calculation, first_number, second_number)
puts "The answer is #{answer}"
puts "Type 1 to run another calcution or 2 to end: "
run_calculator = gets.to_i
end
end
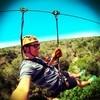
thomascawthorn
22,986 PointsLol I literally couldn't help myself. The above code operates as you want it to - I've just added to_i to the gets fucntion.
gets means get string, so if you're relying on a number you need to convert to an integer (to_i)
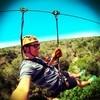
thomascawthorn
22,986 PointsAre you missing an end statement for either of the if statements in "def calculate_answer(operator, a, b)" and "def request_calculation_type"?
def calculate_answer(operator, a, b)
if operator == "add"
return result= a + b
elsif operator == "subtract"
return result = a - b
elsif operator == "multiply"
return result = a * b
elsif operator == "divide"
return result = a / b
#missing end?
end

Daniel Cunningham
21,109 Pointsrequest_calculation_type and calculate_answer need an additional 'end' (1 to end the method, and 1 for the if statements). Additionally, you'll want to add puts or print lines in front of some of your statements (see below). Those lines are not coming up before the gets method is activated.
def greeting puts "Hello! Please type your name: " name = gets
puts "It is nice to meet you #{name}. I am a simple calculator application. \n I can add, subtract, multiply, and divide.\n"
return name
end

Adrian Cunanan
Front End Web Development Techdegree Student 9,996 PointsYeah that was it! gets.to_i
Thanks Tom!!!
Here is the edited code:
# This method greets the user on start up and request their name
# It returns their name
def greeting
puts "Hello! Please type your name: "
name = gets
puts "It is nice to meet you #{name}"
puts "I am a simple calculator application. I can add, subtract, multiply, and divide."
return name
end
# This method ask the user what type of calculation they would like to perform
# It returns the operation or an error for erroneous entry
def request_calculation_type
puts "Type 1 to add, 2 to subtract, 3 to multiply, or 4 to divide two numbers: "
operation_selection = gets.to_i
if operation_selection == 1
return "add"
elsif operation_selection == 2
return "subtract"
elsif operation_selection == 3
return "multiply"
elsif operation_selection == 4
return "divide"
else
return "error"
end
end
# This method performs the requested calculation
# It returns the result of the calculation
def calculate_answer(operator, a, b)
if operator == "add"
return result= a + b
elsif operator == "subtract"
return result = a - b
elsif operator == "multiply"
return result = a * b
elsif operator == "divide"
return result = a / b
end
end
name = greeting
run_calculator = 1
while run_calculator == 1
current_calculation = request_calculation_type()
if current_calculation == "error"
puts "I do not understand this type of calculation... Can we try again?"
else
puts "What is the first number you would you like to #{current_calculation}: "
first_number = gets.to_i
puts "What is the second number you would like to #{current_calculation}: "
second_number = gets.to_i
answer = calculate_answer(current_calculation, first_number, second_number)
puts "The answer is #{answer}"
puts "Type 1 to run another calculation or 2 to end: "
run_calculator = gets.to_i
if run_calculator != 1
puts "Thanks for working with me to crunch some numbers! See you later ;-)"
end
end
end
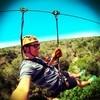
thomascawthorn
22,986 PointsAwesome! If you're as lazy as me, it's handy to remember you don't need to return values with ruby. The last line of the method is automatically returned. You could shorten like so:
return result= a + b
you can just do
a + b
and
return "multiply"
to
"multiply"
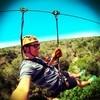
thomascawthorn
22,986 PointsHave you looked at both "def calculate_answer(operator, a, b)" and "def request_calculation_type"? *

Adrian Cunanan
Front End Web Development Techdegree Student 9,996 PointsYeah i realized that after i wrote that last comment that I then deleted after I read your suggestion for both methods ;-)

Adrian Cunanan
Front End Web Development Techdegree Student 9,996 PointsThanks Tom! That resolved the error.
However, the calculator is not working. None of the puts statements seem to output.
Am I calling the methods incorrectly?
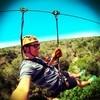
thomascawthorn
22,986 PointsYour "gets" Syntax needs to be more like: (or atleast - this worked for me)
puts "What is your name: "
name = gets
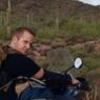
Kevin Naegele
10,868 Pointsi would like to see this app when it is done.

Adrian Cunanan
Front End Web Development Techdegree Student 9,996 PointsYou can add the code in my last comment to a new Workspace. Nothing fancy... It's ALIVE!!!

Adrian Cunanan
Front End Web Development Techdegree Student 9,996 PointsHere is a better version with formatting that makes the program easier to read:
# This method greets the user on start up and request their name
# It returns their name
def greeting
puts "Hello! Please type your name: "
name = gets
puts "\n" + "It is nice to meet you #{name}"
puts "\n" + "I am a simple calculator application. I can add, subtract, multiply, and divide."
return name
end
# This method ask the user what type of calculation they would like to perform
# It returns the operation or an error for erroneous entry
def request_calculation_type
puts "\n" + "Type 1 to add, 2 to subtract, 3 to multiply, or 4 to divide two numbers: "
operation_selection = gets.to_i
if operation_selection == 1
return "add"
elsif operation_selection == 2
return "subtract"
elsif operation_selection == 3
return "multiply"
elsif operation_selection == 4
return "divide"
else
return "error"
end
end
# This method performs the requested calculation
# It returns the result of the calculation
def calculate_answer(operator, a, b)
if operator == "add"
return a + b
elsif operator == "subtract"
return a - b
elsif operator == "multiply"
return a * b
elsif operator == "divide"
return a / b
end
end
name = greeting
run_calculator = 1
while run_calculator == 1
current_calculation = request_calculation_type()
if current_calculation == "error"
puts "\n" + "I do not understand this type of calculation... Can we try again?"
else
puts "\n" + "What is the first number you would you like to #{current_calculation}: "
first_number = gets.to_i
puts "\n" + "What is the second number you would like to #{current_calculation}: "
second_number = gets.to_i
answer = calculate_answer(current_calculation, first_number, second_number)
puts "\n" + "The answer is #{answer}"
puts "\n" + "Type 1 to run another calculation or anything else to end: "
run_calculator = gets.to_i
if run_calculator != 1
puts "\n" + "Thanks for working with me to crunch some numbers! See you later ;-)"
end
end
end
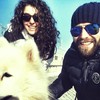
Liviu Tudor
7,061 Pointsjust tried this ! is amazing ! thank you
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsTo save counting, you might want to add where line 69 is with a comment!