Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial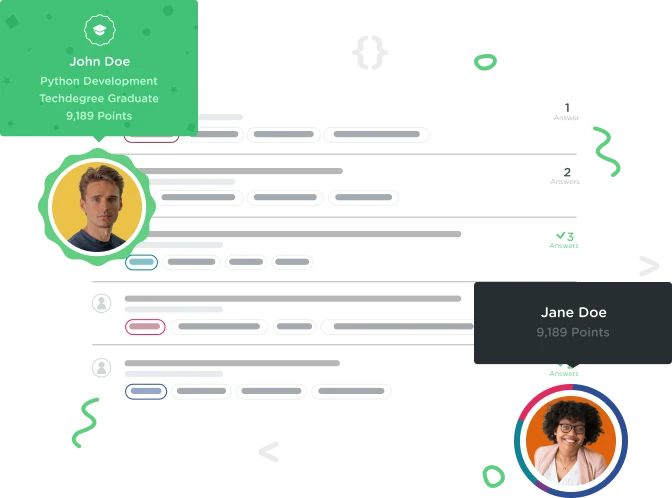

michaelkyllo
11,330 PointsI am trying to change the value of editInput from lable.innerText but it is not working, any thoughts?
//Problem: User interaction doesn't provide desired results.
//Solution: Add interactivty so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder= document.getElementById("completed-tasks"); //completed-tasks
//New Task List Item
var createNewTaskElement = function(taskString) {
//Create List Item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); // checkbox
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("input"); // text
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each element needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editInput.setAttribute("value","value");
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
//Each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//Create a new list item with the text from #new-task:
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
//if the class of the parent is .editMode
if(containsClass) {
//Switch from .editMode
//label text become the input's value
label.innerText = editInput.value;
} else {
//Switch to .editMode
//input value becomes the label's text
editInput.value = label.innerText;
}
//Toggle .editMode on the list item
listItem.classList.toggle("editMode");
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
var newTask = this.parentNode;
var ul = newTask.parentNode;
//Remove the parent list item from the ul
ul.removeChild(newTask);
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Task complete...");
//Append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//Append the task list item to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
var ajaxRequest = function() {
console.log("AJAX request");
}
//Set the click handler to the addTask function
addButton.addEventListener("click", addTask);
addButton.addEventListener("click", ajaxRequest);
//cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++) {
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completedTasksHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++) {
//bind events to list item's children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
<!DOCTYPE html>
<html>
<head>
<title>Todo App</title>
<link href='http://fonts.googleapis.com/css?family=Lato:300,400,700' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" charset="utf-8">
</head>
<body>
<div class="container">
<p>
<label for="new-task">Add Item</label><input id="new-task" type="text"><button>Add</button>
</p>
<h3>Todo</h3>
<ul id="incomplete-tasks">
<li><input type="checkbox"><label>Pay Bills</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
<li class="editMode"><input type="checkbox"><label>Go Shopping</label><input type="text" value="Go Shopping"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
<h3>Completed</h3>
<ul id="completed-tasks">
<li><input type="checkbox" checked><label>See the Doctor</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
</div>
<script type="text/javascript" src="js/app.js"></script>
</body>
</html>
/* Basic Style */
body {
background: #fff;
color: #333;
font-family: Lato, sans-serif;
}
.container {
display: block;
width: 400px;
margin: 100px auto 0;
}
ul {
margin: 0;
padding: 0;
}
li * {
float: left;
}
li, h3 {
clear:both;
list-style:none;
}
input, button {
outline: none;
}
button {
background: none;
border: 0px;
color: #888;
font-size: 15px;
width: 60px;
margin: 10px 0 0;
font-family: Lato, sans-serif;
cursor: pointer;
}
button:hover {
color: #333;
}
/* Heading */
h3,
label[for='new-task'] {
color: #333;
font-weight: 700;
font-size: 15px;
border-bottom: 2px solid #333;
padding: 30px 0 10px;
margin: 0;
text-transform: uppercase;
}
input[type="text"] {
margin: 0;
font-size: 18px;
line-height: 18px;
height: 18px;
padding: 10px;
border: 1px solid #ddd;
background: #fff;
border-radius: 6px;
font-family: Lato, sans-serif;
color: #888;
}
input[type="text"]:focus {
color: #333;
}
/* New Task */
label[for='new-task'] {
display: block;
margin: 0 0 20px;
}
input#new-task {
float: left;
width: 318px;
}
p > button:hover {
color: #0FC57C;
}
/* Task list */
li {
overflow: hidden;
padding: 20px 0;
border-bottom: 1px solid #eee;
}
li > input[type="checkbox"] {
margin: 0 10px;
position: relative;
top: 15px;
}
li > label {
font-size: 18px;
line-height: 40px;
width: 237px;
padding: 0 0 0 11px;
}
li > input[type="text"] {
width: 226px;
}
li > .delete:hover {
color: #CF2323;
}
/* Completed */
#completed-tasks label {
text-decoration: line-through;
color: #888;
}
/* Edit Task */
ul li input[type=text] {
display:none;
}
ul li.editMode input[type=text] {
display:block;
}
ul li.editMode label {
display:none;
}
6 Answers
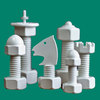
Steven Parker
230,274 PointsYou're looking at the initial attribute value in the Dev Tools.
Try going to the console while that item is highlighted, and type this: $0
It will show you: <input type="text" value="Go Shopping">
original coded value
But if you type this: $0.value
It will show you: "Buy Car"
the current value

michaelkyllo
11,330 PointsThank you, I have spent 10 hours trying to figure our why I couldn't' see changes.
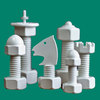
Steven Parker
230,274 PointsI'm not able to replicate the problem.
Now that all the code is here, I tried it and I have no problem switching in and out of edit mode using the button, or changing the text using the input and then having it show up in the label.
Perhaps you could describe the problem in a bit more detail, and/or give explicit steps needed to demonstrate it?
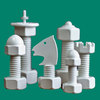
Steven Parker
230,274 PointsIn the screenshot, the highlighted element on the left is inside the folded li
at the top of the right side.
The label with the contents "This should be the input value" is displayed on the bottom of the left side.
Everything looks correct. Did you have the two labels confused with each other?

michaelkyllo
11,330 PointsEssentially, I am under the impression that when I toggle the edit button, the value of the label becomes the value of the input.
essentially
<input type= "text" value="This should be the input value">
Please advise. thank you.
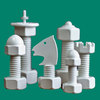
Steven Parker
230,274 PointsYes! That's exactly what the code is designed to do.
And when you toggle it back, the value of the input becomes the label again.

michaelkyllo
11,330 PointsSteven, thanks for your response. This project was from the 'Interactive Web Pages with JavaScript' course. I have updated the question to include the html and CSS code. I would love to see what you find. Thanks again.

michaelkyllo
11,330 PointsSo what I'm trying to do is change the <input type=text>'s value from the label, isn't that what the code is trying to do? Please look at the link to see what I'm referring to. Thanks,

michaelkyllo
11,330 PointsI guess I'm confused because if you go to the "Go Shopping" item and edit it, the value never changes from Go Shopping
See link below, after I've changed the item:
Steven Parker
230,274 PointsSteven Parker
230,274 PointsIt would be helpful for observing the issue if you were to include the HTML (and CSS?) parts of the code.
If this is for a course, a link the course page you were working with would also be helpful.