Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial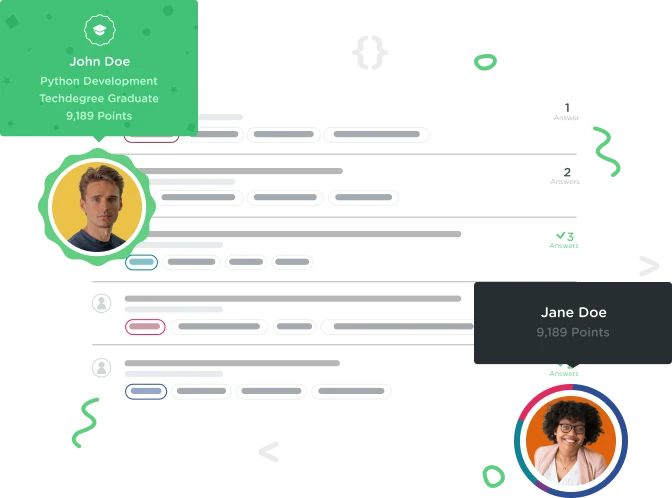

Kenzo Qui
Courses Plus Student 207 PointsI am trying to do the DO loop Challenge and i dont understand can anyone help me?
This is my code, how does this sopposed to work
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
do {
response = console.readLine ("Do you understand do while loops? ");
if (response == "yes") {
console.printf("Try again \n\n");
}
} while (response == "yes");
4 Answers
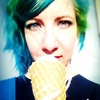
Sara Chicazul
7,475 PointsWhat task are you working on? The question I see reads :
"Now continually prompt the user in a do while loop. The loop should continue running as long as the response is No. Don't forget to declare response outside of the do while loop."
You're looping so long as the answer is yes. Do you have a different challenge?

Dimitri Coukos
2,819 PointsThe loop works like this :
- The loop enters into the do Statement and asks "Do you understand do while loops" to which it expects a reply. ReadLine reads a single string of text which begins when you begin typing on the console, and ends when you hit 'enter'.
- If the reply is yes, then the loop starts again and asks the same question.
- If the reply is no, then you exit the loop and continue after the while statement.
However, you should know that although I'm not an expert in java, as far as I can tell the readline method doesn't take any parameters and only reads. Therefore, you should break that command up into a println("Do you understand while loops") method and then your readLine. Hope this helps!
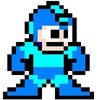
Robert Richey
Courses Plus Student 16,352 PointsHi Kenzo,
That's a really good attempt!
So, it looks like you're having some trouble with task 2.
Now continually prompt the user in a do while loop. The loop should continue running as long as the response is No. Don't forget to declare response outside of the do while loop.
For this part, we don't need any conditional statements within the loop. Also, this do/while loop needs to keep looping while the response is No.
// this loop will run at least once and keep looping while response is "yes"
String response;
do {
response = console.readLine ("Do you understand do while loops? ");
} while (response == "yes");
Paul Rodrigue has a great suggestion to use the .equals()
method on a string object when comparing their values. The double equals operator (==
), when used for comparing strings, compares if both objects are exactly the same - not if they contain the same content. Also, an even better method to use is .equalsIgnoreCase()
.
// this loop will run at least once and keep looping while response is "no"
String response;
do {
response = console.readLine ("Do you understand do while loops? ");
} while (response.equalsIgnoreCase("no"));
By using .equalsIgnoreCase()
, response can be any of "no", "No", "NO", "nO" and still continue the loop.
Hope this helps,
Cheers

Paul Rodrigue
9,420 PointsFor strings you should use the .equals method.
if(someString.equals("other string"){ ...