Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial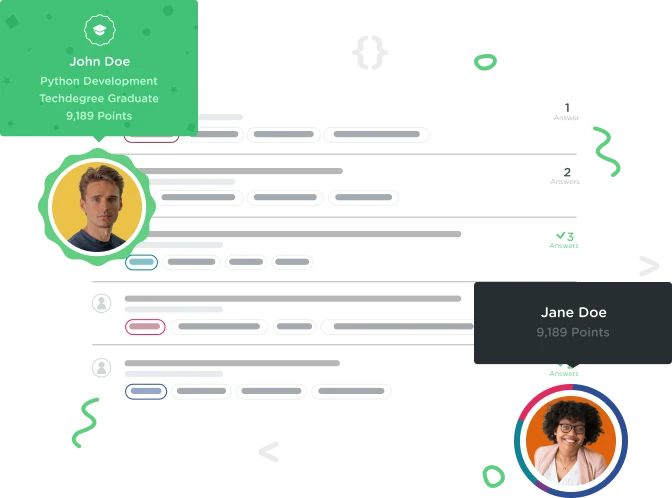
martin chibwe
3,901 PointsI am trying to limit the number of text the user can enter
Use the UITextViewDelegate protocol to limit the number of characters in an entry’s body to two hundred. Also use the protocol to implement a counter to let the user know how many characters have been used so far.
-
(BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text{
if (textView.text.length + text.length <= 200) { NSLog(@"you have reached the max word allowd"); }else if (textView.text.length + text.length <= 0) { NSLog(@"enter somthing please"); return NO; } return YES;
2 Answers

kerdeseverin
9,688 PointsHope this helps ( saw your other post)
To limit the characters to any number try this
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string{
if ([self.theTextField.text length] >= 200) {
return NO;
}
else {
return YES;
}
}
Make sure that you set the delegate of the UITextfield to self and conform to the delegate.
yourTextfield.delegate = self;
and to conform
@interface YourClassViewController ()<UITextFieldDelegate>
If you want to show the user the number of characters left you can use the same [self.theTextField.text length] and update a label or something, elsewhere.
martin chibwe
3,901 Pointsthat helped thanks