Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial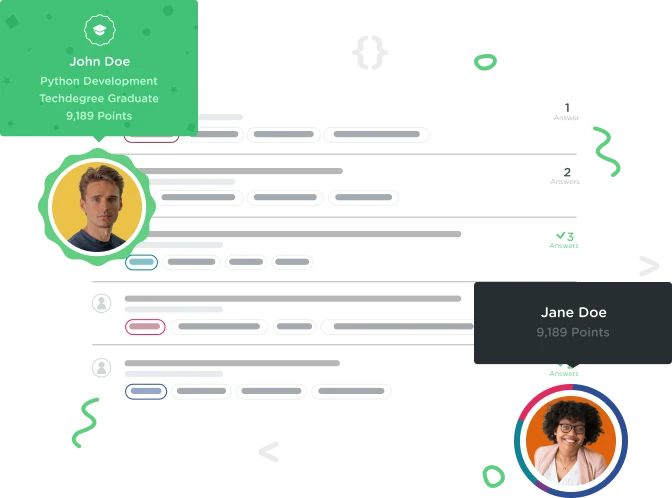

Dare; Rivera
2,297 PointsI am trying to understand why I get this error Exception in thread "main" java.lang.NullPointerException
interface NavyAviation//interface with two abstract methods
{
public void NavyAviation();
public void SearchAndRescue();
}
public class Aircrew//superclass
{
public void Aircrew()
{
System.out.println("Naval Rescue Swimmer(SAR) is the best rate in the Navy");
}
}
public class AircrewMember//super class with data members, accessor, mutator, and toString methods
{
private String name;
private Date ad;
public AircrewMember()
{
name=" ";
ad=null;
}
public AircrewMember(String name, Date ad)
{
this.name=name;
this.ad=ad;
}
public void setName(String name)
{
this.name=name;
}
public String getName()
{
return name;
}
public String toString()
{
String str="AW's name is " + name + "\nAW's arrival date is: "+ ad.toString();
return str;
}
}
public class AWInfo extends AircrewMember/*subclass with data members,
accessor, mutator, and toString methods*/
{
private int rate;
final static int AWR_RATE=1;
final static int AWS_RATE=2;
public static final int MAX_RATE=2;
private AircrewMember Aw;
public AWInfo()
{
rate=0;
/*shift=0;*/
Aw=null;
}
public AWInfo(int rate, AircrewMember Aw)//method overloading
{
this.rate=rate;
this.Aw=Aw;
}
public void rate()
{
rate();
}
public void rate(int rating)throws InvalidRate//exception handeling
{
if(rating<0 || rating>MAX_RATE)
/*(rating != "AWR" || rating != "AWS")*/
throw new InvalidRate(rating);
rate=rating;
}
/*public void shift()
{
shift();
}
public void shift(int workShift) throws InvalidShift//exception handeling
{
if(workShift<0 || workShift>MAX_SHIFT)
throw new InvalidShift(workShift);
shift=workShift;
}*/
public int getRate()
{return rate;}
/*public int getShfit()
{return shift;}*/
public String toString()//method overriding
{
String str= super.toString();
str+="\nRate: ";
if(rate==AWR_RATE)
str+= "AWR";
if(rate==AWS_RATE)
str+="AWS";
return str;
}
}
public class ProductionWorker extends Employee
{
final static int DAY_SHIFT=1;
final static int NIGHT_SHIFT=2;
public static final int MAX_SHIFT=2;
private int shift;
private double payRate;
public ProductionWorker()
{
super();
shift=0;
payRate=8.50;
}
public ProductionWorker(String name, Date hd/*, int number, int shift, double payRate*/)
{
super(name, /*number,*/ hd);
/*this.shift=shift;
this.payRate=payRate;*/
}
public void shift()
{
shift();
}
public void shift(int workShift) throws InvalidShift
{
if(workShift<0 || workShift>MAX_SHIFT)
throw new InvalidShift(workShift);
shift=workShift;
}
public void payRate()
{
payRate();
}
public void payRate(double newPay)throws InvalidPayRate
{
if(newPay<payRate)
throw new InvalidPayRate(newPay);
payRate=newPay;
}
public int getShift()
{
return shift;
}
public double getPayRate()
{
return payRate;
}
public String toString()
{
String str= super.toString();
str +="\nShift: ";
if (shift == DAY_SHIFT)
str += "Day";
else if (shift == NIGHT_SHIFT)
str += "Night";
else
str += "Invalid Shift Number";
str += "\nHourly Pay Rate: ";
return str;
}
}
import java.util.Scanner;
import java.io.*;
public class FreedomFighters extends Aircrew implements NavyAviation
{
public void NavyAviation()
{System.out.println("Enlisted Naval Aviaton");}
public void SearchAndRescue()
{System.out.println("Search and Rescue rates holds the only real AW's");}
public void Aircrew()
{System.out.println("This is for all AWRs and AWSs, all other are not welcomed");}
public static void main(String[] args)
{
FreedomFighters ff1=new FreedomFighters();
ff1.NavyAviation();
ff1.SearchAndRescue();
ff1.Aircrew();
Scanner keyboard=new Scanner(System.in);
int payGrade, Ar,rate, idNumber, ws, day, month, year;
String name;
AWInfo f1=new AWInfo();
try
{
System.out.println("Enter 1 for AWR or 2 for AWS: ");
Ar=keyboard.nextInt();
f1.rate(Ar);}
catch(InvalidRate e){
System.out.println(e.getMessage());
System.exit(0);}
/*try{
System.out.println("Enter 1 for morning shift or 2 for evening shift");
ws=keyboard.nextInt();
f1.shift(ws);
}
catch(InvalidShift e)
{System.out.println(e.getMessage());}*/
System.out.println();
keyboard.nextLine();
System.out.print("Enter new AW's name: ");
name = keyboard.nextLine();
System.out.print("Enter AW's arrival day: ");
day = keyboard.nextInt();
System.out.print("Enter AW's arrival month: ");
month = keyboard.nextInt();
System.out.print("Enter AW's arrival year: ");
year = keyboard.nextInt();
Date ad = new Date(day, month, year);
AircrewMember a1 = new AircrewMember(name, ad);//aggregation
System.out.println(f1.toString());//aggregation
}
}