Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial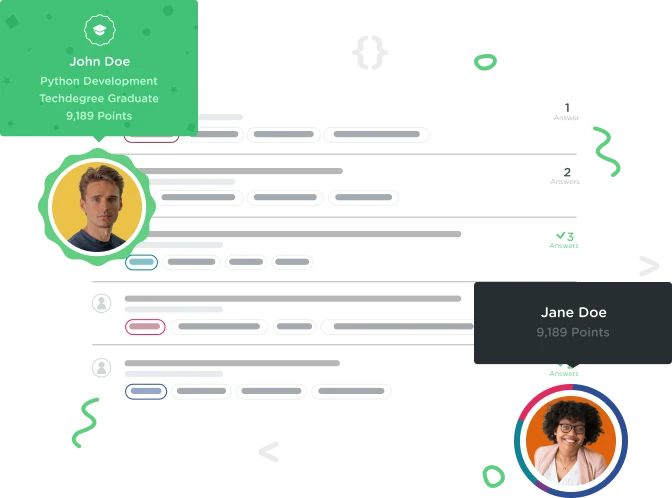

Paul Zutrau
7,423 PointsI am unable to complete question on java arrays.
The videos show functions using the console, not a java doc. The question looks easy but after trying several times reviewing the video I can't place the correct code. Any help is appreciated. Thanks Paul
In the class TeachersExamples there are two methods. One shows a longer version of how to create an array. Your task is to create the array in a shorter literal form in the method getTeachersAsArrayLiteral, replacing the null assignment. public class TeachersExample { public String[] getTeachersInArrayLongForm() { String[] teachers = new String[3]; teachers[0] = "Jay"; teachers[1] = "Dave"; teachers[2] = "James"; return teachers; } public String[] getTeachersAsArrayLiteral() { // TODO: Replace null with an array literal that matches the long form above String[] teachers = null; return teachers; } }
public class TeachersExample {
public String[] getTeachersInArrayLongForm() {
String[] teachers = new String[3];
teachers[0] = "Jay";
teachers[1] = "Dave";
teachers[2] = "James";
return teachers;
}
public String[] getTeachersAsArrayLiteral() {
// TODO: Replace null with an array literal that matches the long form above
String[] teachers = null;
return teachers;
}
}
2 Answers

eotfofiw
Python Development Techdegree Student 824 PointsHello Paul!
The first method getTeachersInArrayLongForm
is showing you how you can create an array with a certain length. It then proceeds to add strings to that array in each "slot" that has been created in the array.
public String[] getTeachersInArrayLongForm() {
// Create an array of strings, with length 3.
String[] teachers = new String[3];
// We now have a box that looks like this: [empty, empty, empty]
// Take the first empty slot and put "Jay" in there
teachers[0] = "Jay";
// Take the second empty slot and put "Dave" in there
teachers[1] = "Dave";
// Take the third empty slot and put "James" in there
teachers[2] = "James";
return teachers;
}
To pass the solution, we need to have this method create the same array as above, but using the literal notation
public String[] getTeachersAsArrayLiteral() {
// TODO: Replace null with an array literal that matches the long form above
String[] teachers = null;
return teachers;
}
String[] teachers
should stay there. We need that variable to hold the value of the array we need to create, and specify it's type.
null
is currently being assigned to teachers
and that's not right.
There are a couple ways to create an array using literal notation:
// Specify the type in the variable AND specify the type in the assignment
String[] arrayOfStrings = new String[] { "Apples", "Oranges", "Strawberries" };
// Specify the type in the variable, but just rely on the compiler to know, based on the type in the variable, WHAT will be expected. This removed the duplication of String[] on the left and right of the assignment
String[] arrayOfStrings = { "Dogs", "Cats", "Hamsters" }

Paul Zutrau
7,423 Pointsthank you I most appreciate it.