Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial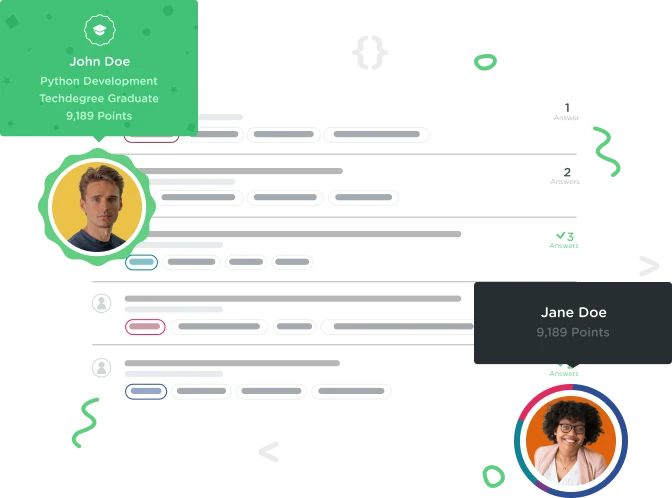
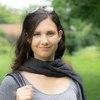
Marta Volpi
3,104 PointsI answered in a different way, but it works! Can I improve it?
So, this is my code:
var message = ''; var student;
for( var i = 0; i < students.length; i += 1){ student = students[i]; for(var prop in student){ if (prop === 'name'){ message = '<h2>' + prop + ': ' + student[prop] + '</h2>'; document.write(message); } else{ message = '<p>' + prop + ': ' + student[prop] + '</p>'; document.write(message); } } }
However, i am not using the function print but I'm just using document.write(). Is this a good practice? How could I include the function print without overwriting the div id='output' any loop of the for?
Hope it makes sense :)
2 Answers
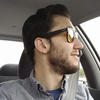
Benjamin Larson
34,055 PointsIf you wanted to combine your solution with Dave's print() function, this would be one way to do it:
var message = "";
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for( var i = 0; i < students.length; i += 1) {
student = students[i];
for(var prop in student) {
if (prop === 'name') {
message += '<h2>' + prop + ': ' + student[prop] + '</h2>';
} else {
message += '<p>' + prop + ': ' + student[prop] + '</p>';
}
}
}
print(message);
In a sense your solution "feels" more elegant by utilizing and printing the property names, but in practice it may have some problems. The more minor problem is that it makes formatting the output difficult, in particular if the property names in the object/array are not labeled exactly how you would want to output them. The more significant problem would be if there were additional properties in the student array that you did not want to output at all. You could filter them out, but the loop would still have to go through each of them and in a very large array with a lot of values, this would be an expensive operation.
But it's still good that you came up with a different solution. There are often different ways to accomplish the same thing, and your way may well be better in some instances.
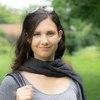
Marta Volpi
3,104 PointsRight, by changing '''message =''' to '''message +=''' I can avoid overwriting the message over and over, but I can't rename the properties or select the ones I want to print from a larger array.
I see your point, this solution is not as flexible as Dave's solution.
Thanks for replying!