Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial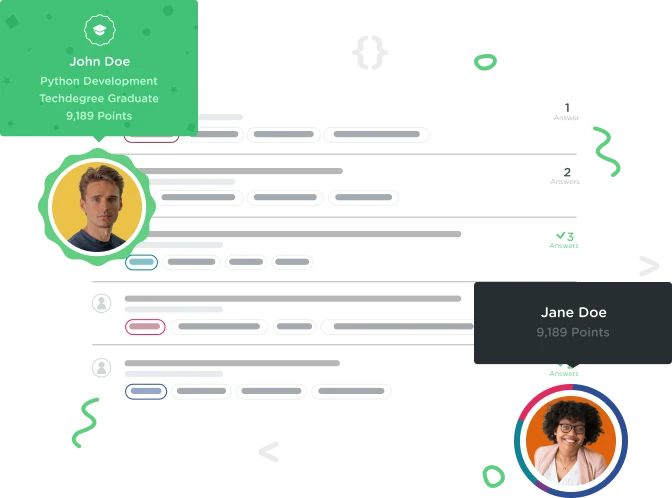
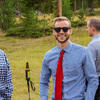
patrick ridd
21,011 PointsI believe I have the answer for part 2, but it still won't compile?
I have ran this code in an xCode playground and it works and outputs exactly how the example text says it should. Despite that, this challenge says it won't compile and doesn't give me any errors in preview. Not sure how to fix this. Thanks.
-Patrick
struct Tag {
let name: String
}
struct Post {
let title: String = "iOSDevelopment"
let author: String = "Apple"
let tag = Tag(name: "swift")
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post()
let postDescription = firstPost.description()
2 Answers

Anjali Pasupathy
28,883 PointsYou've nearly got it. The only thing wrong with your code is you shouldn't instantiate the properties of Post inside the struct. Rather, instantiating the properties of Post should happen when you instantiate a Post object (similar to how in Tag, you instantiate tag.name when you instantiate a Tag object).
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: /*STRING*/, author: /*STRING*/, tag: /*TAG*/)
I hope this helps!
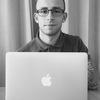
James Knight
4,680 Pointsimport Foundation
struct Tag { let name: String }
struct Post { let title: String = "iOSDevelopment" let author: String = "Apple" let tag = Tag(name: "swift")
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
} let firstPost = Post() let postDescription = firstPost.description()
I have just entered this into the challenge and it has accepted the answer, the only thing that i changed was to import foundation. hope this helps !
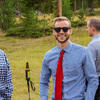
patrick ridd
21,011 PointsI tried to add the "import Foundation" statement and it still didn't work. That's funny that it worked on yours though. Thanks for the feedback, James.
patrick ridd
21,011 Pointspatrick ridd
21,011 PointsThanks Anjali! That cleared it up.
Anjali Pasupathy
28,883 PointsAnjali Pasupathy
28,883 PointsYou're welcome! Glad I could help.