Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial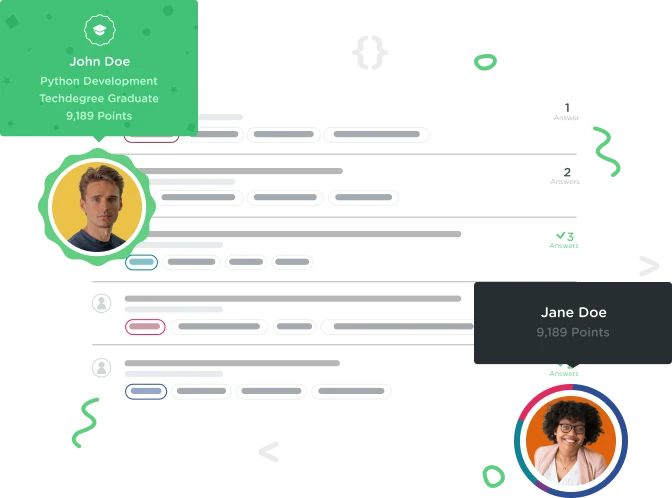

Max Gabriel
11,213 PointsI believe i have to set the initial condition from an || operator to an && operator to ensure both conditions are met.
.
var money = 9;
var today = 'Friday';
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

joelearner
54,915 PointsHi Max,
You're off to a good start! If you remember the way the challenge is worded, the problem with the current code is that the first if statement ("Time to go to the theater") is being returned even though the value for money is only 9. You have fixed the first statement by changing the || to &&. However, if you run the code again, you will return the statement "Time for a movie and dinner". This is because the second statement still has an or(||) condition in place. So as long as it is Friday, the value of 9 may produce an inaccurate statement.
Try changing all of the conditions from || to && to ensure both conditions are met to print the qualifying alert statement.
Then at the 3rd else if, you have:
else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
Here, the alert is saying "It's Friday"... so you should change the !== to === to include Friday as a condition. Then, look again at the value for var money. It is only 9. You will need to include a value range in the same line that will capture a value less than 10 with an && condition. So change that line:
else if ( today === 'Friday' && money <=10 ) {
alert("It's Friday, but I don't have enough money to go out");
Combining these areas together, your completed code should look something like this:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' && money <=10 ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Cheers!
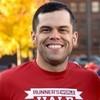
Juan Lopez
Treehouse Project ReviewerSo lets look at each line of your conditionals to see if what is being outputted/alerted is matching the conditional
var money = 9;
var today = 'Friday';
//This one is checking to see if money is greater than or equal to 100 and today is equaling Friday which I guess they are skipping the movie and going big bucks style to the theatre
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
}// The way it is written here you are checking to see if money is greater than or equal to 50 or today is Friday but it does not match the alerted statement since we don't have enough money for both movie and a dinner so the better option would be the "&&"
else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
}
// Once again the way the conditional is written it is checking to see if money is greater than 10 or today is Friday but since we do not have enough money but today is Friday then the conditional would stop here which it should not since we don't have enough money so the better option would once again be the "&&"
else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
}// This one is the conditional does not match because the conditional is checking for it to NOT be Friday. So changing it from a "!==" to a "===" would satisfy that requirement
else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
So with some simple changes we get
var money = 9;
var today = 'Friday';
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}