Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial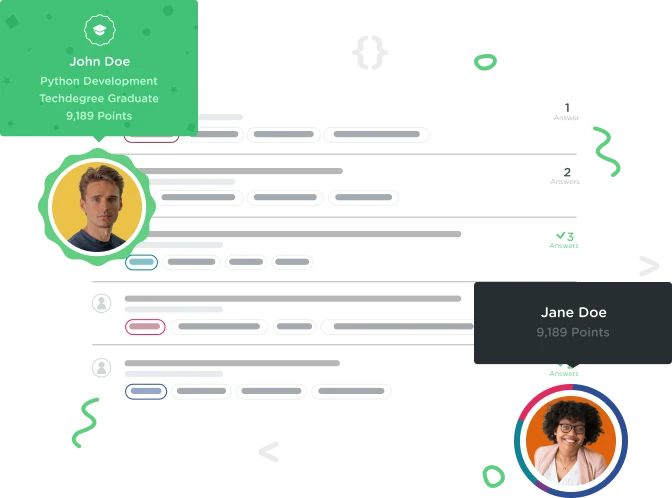

Justin Lopes
614 PointsI believe this is correct but it keeps telling me it is wrong?
I don't understand what I am doing wrong
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
for n in 1...100 {
if (n % 3 == 0) && (n % 5 == 0) {
print("fizzBuzz")
} else if (n % 3 == 0) {
print("Fizz")
} else if (n % 5 == 0) {
print("Buzz")
} else {
print (n)
}
}
// End code
return "\(n)"
}
1 Answer
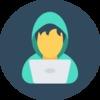
Patrick Marshall
13,508 PointsHi Justin. You're pretty close here. It looks like you've added an unnecessary for loop, causing your code to go through each number from 1 to 100 and print the results. Your function is setup to accept an integer to check against.
Step 3: Change all your print statements to return statements. For example: print("FizzBuzz") becomes return "FizzBuzz". You didn't change your print statements to return statements. So it's not going to return a result to the calling function.
Note: Do not worry about the default case (where the number doesn't match Fizz, Buzz, or FizzBuzz). You really don't need the default else case as a default value is returned if none of the other comparisons equate to tru. But, if you wish to keep it, you need to make sure a string is returned like it is formatted in the last return statement under the end code comment.