Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial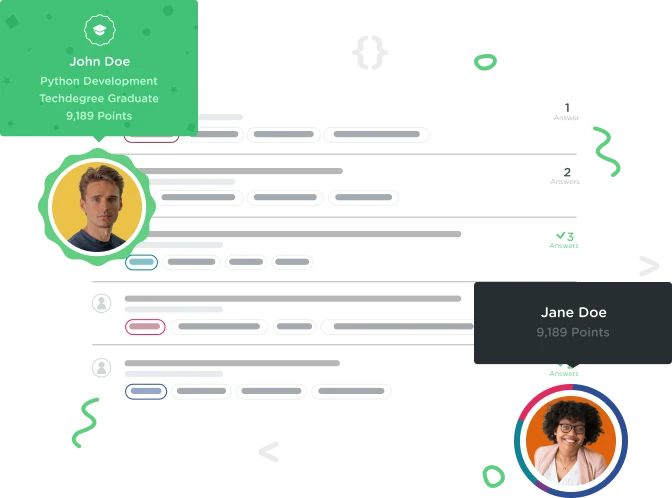

Alexander Gyger
14,237 PointsI came up with a solution which seperates the questionning from the html-build-up. Is this desirable or less so?
Before the solution i came up with a solution with these ideas in mind:
- seperating the questionning and the html-build-up
- storing the answers into the original array (true/false)
- making the input more robust (also adding "skipped" questions)
Now knowing the teacher's solution and comments my further questions are:
- Is is still readable enough, even though I did not use that many variables as Dave?
- Is it ok to alternate the original array?
This is the code:
var corrAnsw = 0;
var answSkipped = 0;
var htmlOutput = '';
var quizList = [
['What was the profession of the famous Malevich?', 'painter'],
['How many cantons does Switzerland have?', '26'],
['Are Java and JavaScript the same?', 'no'],
['What is the capital of Germany?', 'berlin'],
['What was the keyword for the activation of the audio interface of the star ships in the Star Trek Series?', 'computer']
];
function print(message) {
document.write(message);
}
function runQuiz(passArray) {
for( i = 0; i < passArray.length; i += 1) {
var currentQuestion = '';
while (currentQuestion === ''){
currentQuestion = prompt(passArray[i][0]);
}
//4 input states: empty, cancelled(skipped), wrong, correct
if (currentQuestion != null) {
if (currentQuestion.toLowerCase() === passArray[i][1]){
corrAnsw += 1;
//console.log('corrAnsw: ' + corrAnsw);
passArray[i].push(true);
} else {
passArray[i].push(false);
}
} else {
passArray[i].push(false);
answSkipped += 1;
}
//console.log('passArray[i][2]: ' + passArray[i][2]);
}
}
function createList(passPhrase) {
htmlOutput += '<h2>' + passPhrase + '</h2><ol>';
for(i = 0; i < quizList.length; i += 1) {
//list all correctly answered questions
if (quizList[i][2]){
htmlOutput += '<li>' + quizList[i][0] + '</li>';
}
}
htmlOutput += '</ol>';
}
function createWrong(passPhrase) {
htmlOutput += '<h2>' + passPhrase + '</h2><ol>';
for(i = 0; i < quizList.length; i += 1){
//list all wrongly answered questions + display solutions
if (!quizList[i][2]){
htmlOutput += '<li>' + quizList[i][0] + ' The right answer was: ' + quizList[i][1] + '. </li>';
}
}
htmlOutput += '</ol>';
}
//Running the quiz, saves answers (true/false) to the "quizList"-array
runQuiz(quizList);
//console.log(quizList);
//Building up HTML-output after the quiz
htmlOutput += '<h2>You got ' + corrAnsw + ' of a total of ' + quizList.length + ' question(s) right.</h2>';
//Displaying skipped
if(answSkipped > 0) {
htmlOutput += '<h2>You skipped ' + answSkipped + ' question(s).</h2>';
}
//A - 'You got all question(s) correct:'
//B - 'You got all question(s) wrong:'
//C - 'You got these question(s) correct:'
// 'You got these question(s) wrong:'
if (corrAnsw === quizList.length) {
createList('You got all questions correct:');
} else if (corrAnsw === 0) {
createWrong('You got all questions wrong:');
} else {
createList('You got these question(s) correct:');
createWrong('You got these question(s) wrong:');
}
print(htmlOutput);